Google Lens is a visual search tool that allows users to upload images to find similar or exact matches of items and products. By analyzing the visual content of the uploaded image, Google Lens can identify and compare it with its online image database. This capability is especially valuable for discovering visually similar products, whether clothing, furniture, or artwork.
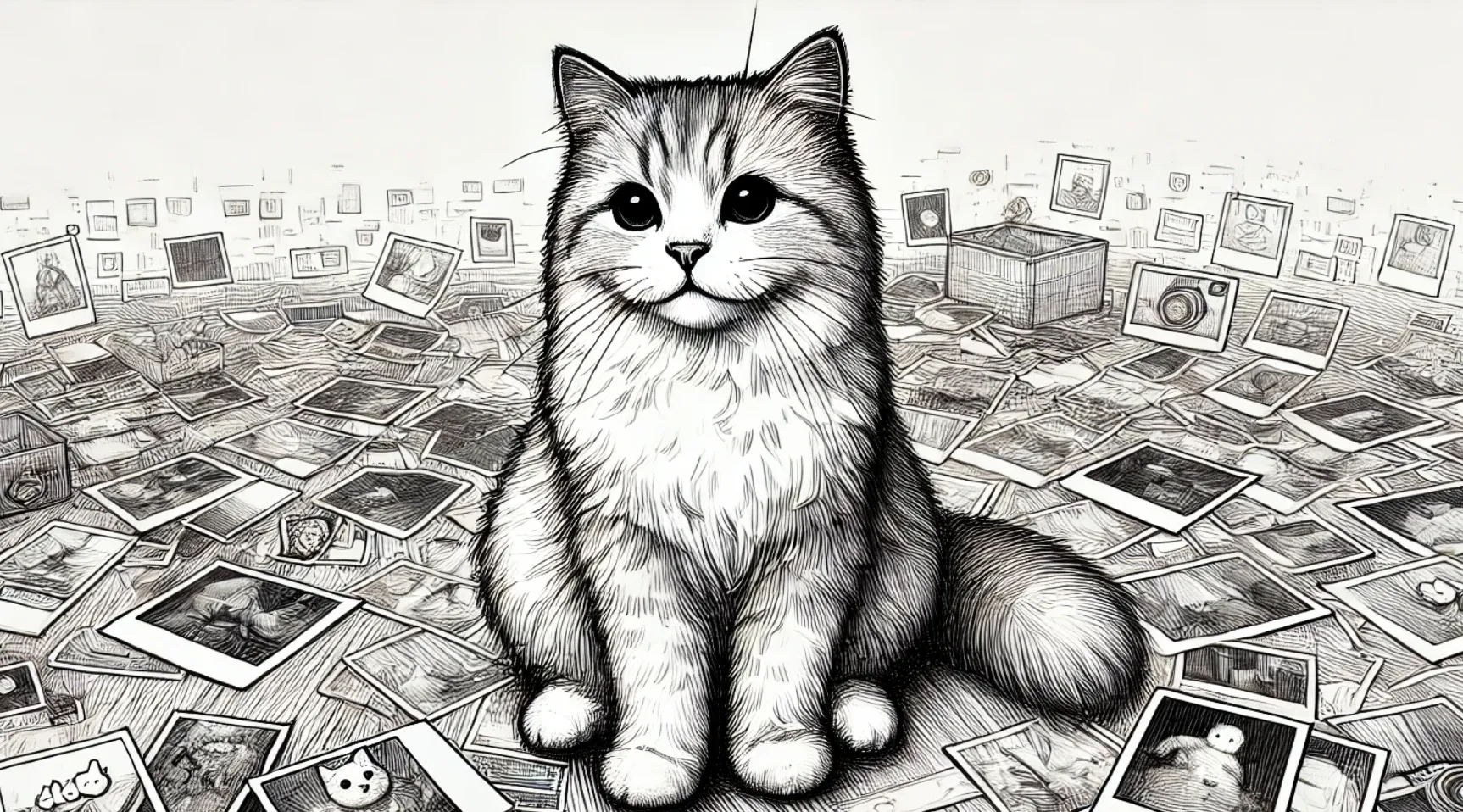
Why scrape Google Lens?
If you need to search for many images, doing so one by one could be time-consuming. Using our Google Lens API, you'll be able to do it programmatically.
Preparation
You can use a simple GET request or a dedicated SDK based on your programming language, including Python, Javascript, and more. In this post, we'll use the GET request method in Python.
Preparation for accessing SerpApi API
- Register at serpapi.com for free to get your SerpApi Api Key.
- Create a new
main.py
file - Install requests with
pip install requests
Here is what the basic setup looks like
import requests
SERPAPI_API_KEY = "YOUR_REAL_SERPAPI_API_KEY"
params = {
"api_key": SERPAPI_API_KEY, #replace with your real API Key
# soon
}
search = requests.get("https://serpapi.com/search", params=params)
response = search.json()
print(response)
With these few lines of code, we can access all of the search engines available at SerpApi, including Google Lens. We'll need to adjust the parameters based on our needs.
Scrape Google Lens using Python
The Google Lens API requires a valid image link that is available publicly. We'll use this link to put in the url
. For example, I have this image here: https://i.imgur.com/HBrB8p0.png. Here is how to search for this image on Google Lens.
import requests
SERPAPI_API_KEY = "YOUR_REAL_SERPAPI_API_KEY"
params = {
"api_key": SERPAPI_API_KEY, #replace with your real API Key
"engine": "google_lens",
"url": "https://i.imgur.com/HBrB8p0.png",
}
search = requests.get("https://serpapi.com/search", params=params)
response = search.json()
print(response)
You should be able to see the response from our API in the console.
Inspect page
If you want to have a better view of the response, you can grab the search_metadata > id on the response and access this page: https://serpapi.com/searches/{the search ID you got}/inspect
Be sure to replace it with your search ID. Here is what my search looks like:
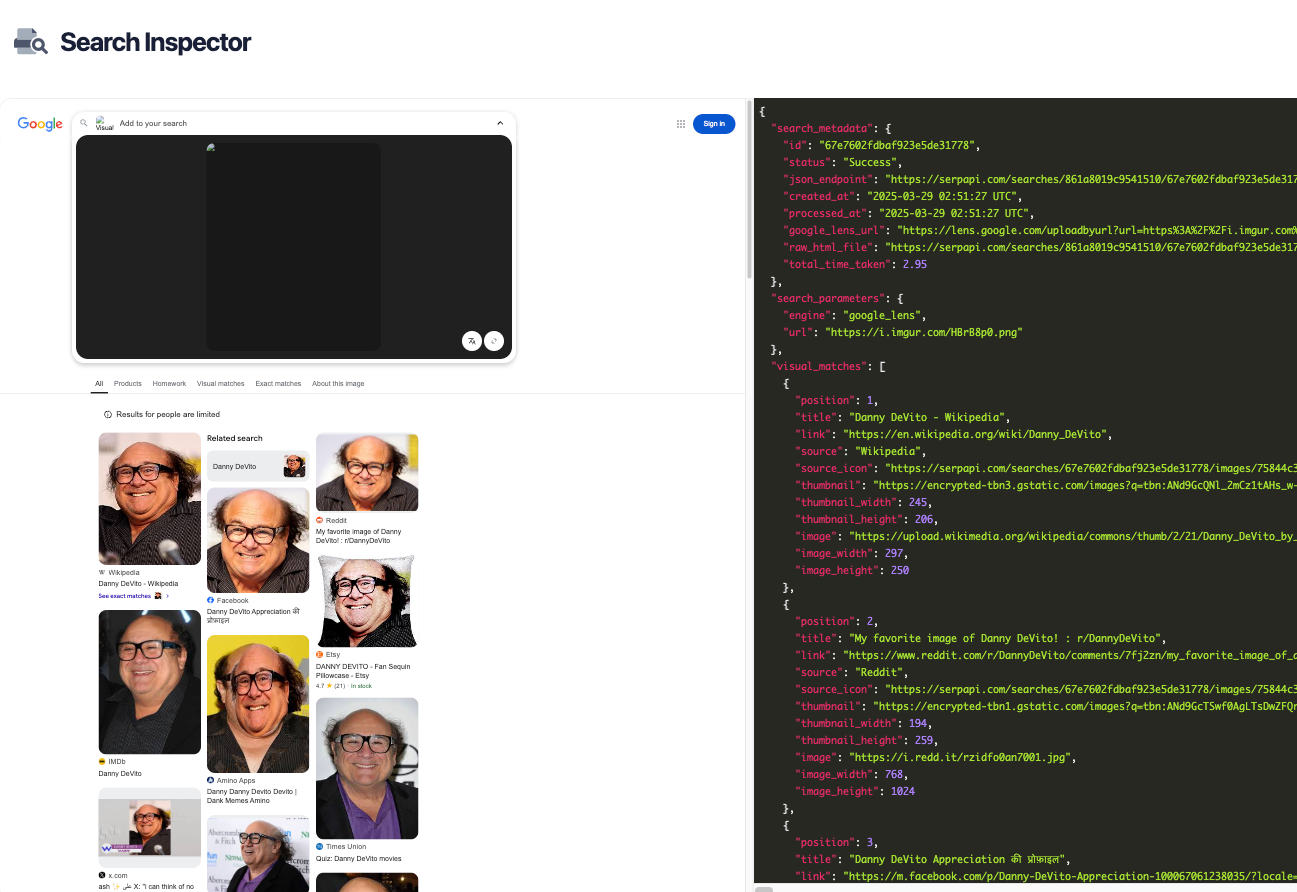
On the left side, you can see the HTML version; you can click on any of the images, and it'll scroll the response to the correct position on the right side. As you can see, we provide the initial search results in this format:
{
"position": -,
"title": "Danny DeVito",
"link": "https://www.imdb.com/media/rm2640023040/nm0000362",
"source": "IMDb",
"source_icon":
"https://serpapi.com/searches/67e7602fdbaf923e5de31778/images/75844c3641e5f327d71f86b6cf522521ed2f4d4f5037c079d22dece044a1b073.png",
"thumbnail":
"https://encrypted-tbn2.gstatic.com/images?q=tbn:ANd9GcRTh4Zy6emTiteMDJoE7d29Hq1x_B78Ot_wv3CiUTmdgpm3G6MH",
"thumbnail_width": 266,
"thumbnail_height": 189,
"image": "https://m.media-amazon.com/images/M/MV5BNzc0NjMxMDM5MV5BMl5BanBnXkFtZTcwMTUyNjYwMw@@._V1_.jpg",
"image_width": 400,
"image_height": 285
},
You can do anything you want with the data available.
Scraping different tabs
On Google Lens, you can navigate the menu at the top to see different results depending on which menu you click.
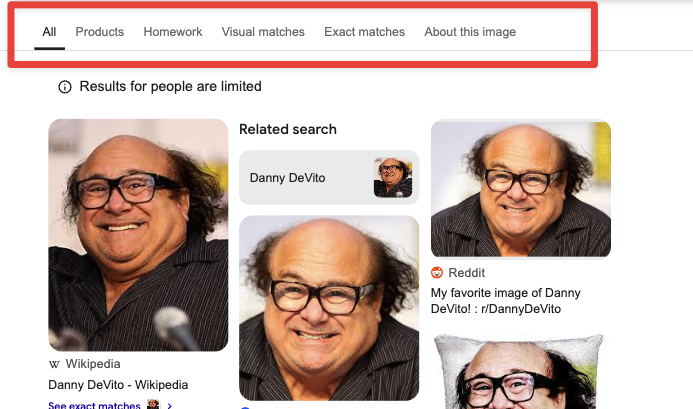
To do this with our API, you need to perform another request, but this time, include the relevant token. The tokens for each menu are unique; it's available on the initial response of your search:
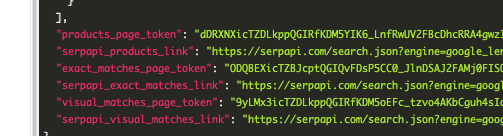
Scrape the exact matches tab from Google Lens
Now, let's adjust the param to access the specific tab. This time, we're going for exact matches
tab.
params = {
"api_key": SERPAPI_API_KEY, #replace with your real API Key
"engine": "google_lens",
"country": "US", # You can adjust to specific country
"page_token": "token_from_initial_response->exact_matches_page_token"
}
Please note that we shouldn't add url
when accessing tab-specific results.
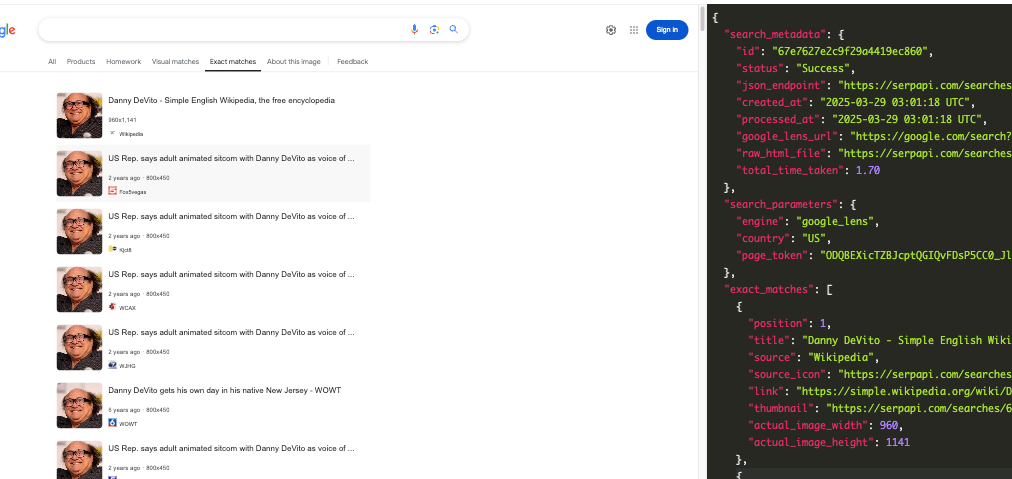
Scrape the visual matches tab from Google Lens
Similar to the previous method, this time, you can add the value from visual_matches_page_token
for the page_token
paramter.
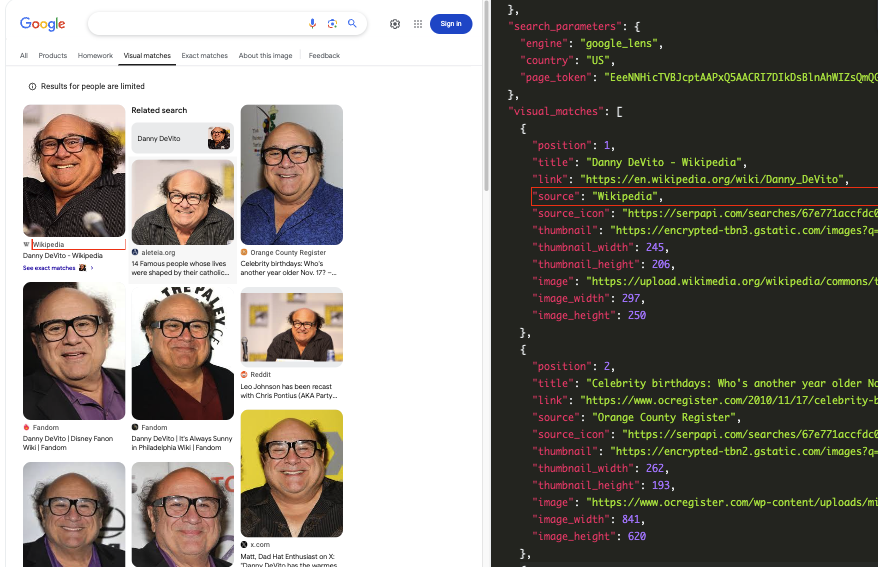
Scrape product tab from Google Lens
Again, we'll use the same method. This time we put the products_page_token
as the page_token
value to scrape the search results from Products
tab.
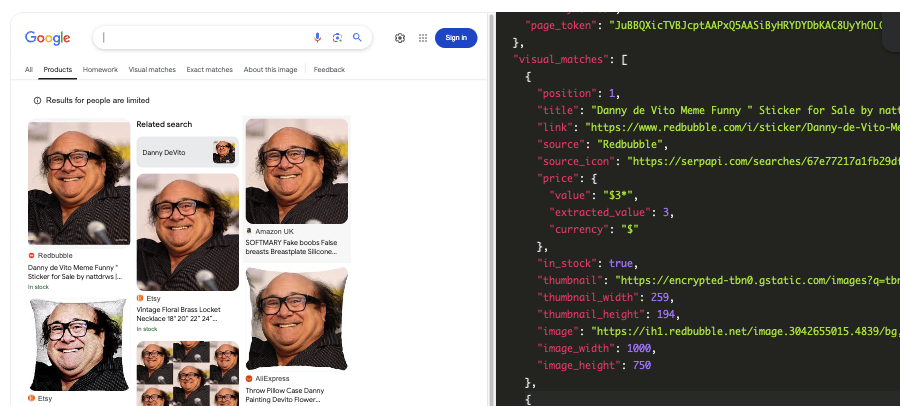
As you can see, the products tab can include additional information for an online shop like link
, source
, or price
. It's perfect if you're looking for specific product information.