If you have a URL of an image and want to download it to your local computer programmatically, you're coming to the right place! We'll see how to do this programmatically using Python and NodeJS.
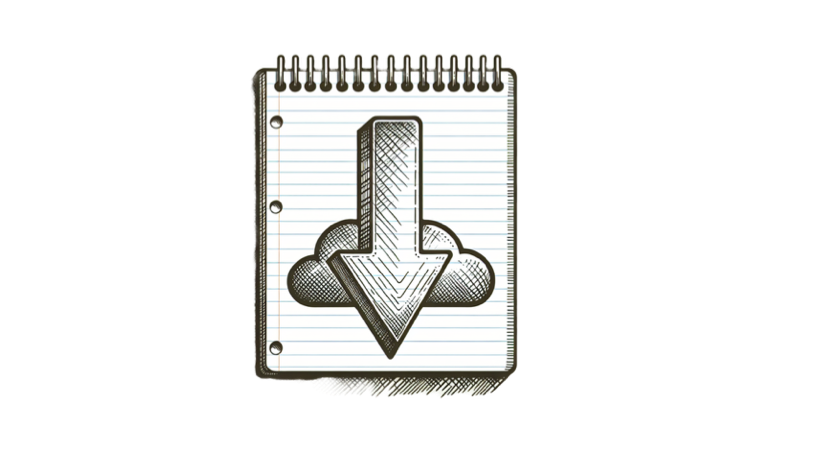
Download images from a URL using Python
Here is the step-by-step and a code sample of how to download an image from a website with Python.
Here is the full code snippet:
import requests
# URL of the image
url = 'YOUR IMAGE URL'
response = requests.get(url)
if response.status_code != 200:
print("Failed to download image!")
exit()
filename = url.split('/')[-1] # You can name the file as you want
with open(filename, 'wb') as file:
file.write(response.content)
print("Image downloaded successfully!")
Here is the step-by-step explanation. Make sure to create a new file with .py
extension to run your code:
Step 1: Import request
We'll use the request library to access any URL from the internet.
import request
# URL of the image
url = 'YOUR IMAGE URL'
response = requests.get(url)
Step 2: Check if there's any issue with the link
Check if the status code is not 200
if response.status_code != 200:
print("Failed to download image!")
exit()
Step 3: Save the image file
Let's store this file using the open method to write or create the file. You can name it whatever you want. In this case, I'm using the original name of the file/url.
filename = url.split('/')[-1] # You can name the file as you want
with open(filename, 'wb') as file:
file.write(response.content)
print("Image downloaded successfully!")
Download multiple images from URLs using Python
Now, let's take a look at how to download multiple images from multiple URLs. The idea is the same as before. We just need to loop the image URLs and place them in a directory.
import requests
import os
# List of image URLs
image_urls = [
'https://imageurl.com/1.jpg',
'https://imageurl.com/2.jpg'
]
# You can name the dir whatever you want
save_dir = 'downloaded_images'
# Validate that the directory exists
if not os.path.exists(save_dir):
os.makedirs(save_dir)
# Function to download an image
def download_image(url, save_path):
try:
response = requests.get(url)
response.raise_for_status() # Raises an error on a bad status
with open(save_path, 'wb') as file:
file.write(response.content)
print(f"Image saved to {save_path}")
except requests.RequestException as e:
print(f"Failed to download {url}: {e}")
# Download each image
for i, url in enumerate(image_urls):
filename = f"image_{i+1}.jpg" # Feel free to replace each of the name
save_path = os.path.join(save_dir, filename)
download_image(url, save_path)
Download image using Javascript (NodeJS)
Here is the full source code on how download a single image from a URL using NodeJS:
const axios = require('axios');
const fs = require('fs');
const path = require('path');
// URL of the image to download
const imageUrl = 'https://imageurl.com/image.jpeg';
// Where to save the image
const savePath = path.resolve(__dirname, 'downloaded_image.jpg');
const downloadImage = async (url, filepath) => {
try {
const response = await axios({
url,
method: 'GET',
responseType: 'stream'
});
const writer = fs.createWriteStream(filepath);
response.data.pipe(writer);
return new Promise((resolve, reject) => {
writer.on('finish', resolve);
writer.on('error', reject);
});
} catch (error) {
console.error('Error downloading the image:', error);
}
};
downloadImage(imageUrl, savePath).then(() => {
console.log('Image downloaded successfully!');
});
Here is the Step-by-Step explanation:
Step 1: Prepare a new project
Create a new file, name it whatever you want. Then, initialize a new npm project on the same directory.
npm init -y
Step 2: Install Axios package
We'll use Axios to access the URL. You can use any other HTTP library like fetch, needle, etc.
npm i axios --save
Step 3: Prepare image source and destination
To make it tidy, let's store the source and destination file name on a variable.
// URL of the image to download
const imageUrl = 'https://imageurl.com/image.jpeg';
// Where to save the image
const savePath = path.resolve(__dirname, 'downloaded_image.jpg');
Step 4: Download image method
Now it is the important part. Let's create the download image method using createWriteStream
from fs
built-in module.
const downloadImage = async (url, filepath) => {
try {
const response = await axios({
url,
method: 'GET',
responseType: 'stream'
});
const writer = fs.createWriteStream(filepath);
response.data.pipe(writer);
return new Promise((resolve, reject) => {
writer.on('finish', resolve);
writer.on('error', reject);
});
} catch (error) {
console.error('Error downloading the image:', error);
}
};
downloadImage(imageUrl, savePath).then(() => {
console.log('Image downloaded successfully!');
});
Download multiple images using Javascript
Now, let's see how to download multiple images from different URLs using Nodejs (Javascript):
const axios = require('axios');
const fs = require('fs');
const path = require('path');
const imageUrls = [
'https://imageurl.com/1.jpg',
'https://imageurl.com/2.jpg'
];
// Where to store the downloaded images
const downloadFolder = path.resolve(__dirname, 'downloaded_images');
// Make sure it exists
if (!fs.existsSync(downloadFolder)) {
fs.mkdirSync(downloadFolder);
}
const downloadImage = async (url, filepath) => {
try {
const response = await axios({
url,
method: 'GET',
responseType: 'stream'
});
const writer = fs.createWriteStream(filepath);
response.data.pipe(writer);
return new Promise((resolve, reject) => {
writer.on('finish', resolve);
writer.on('error', reject);
});
} catch (error) {
console.error(`Error downloading the image from ${url}:`, error);
}
};
const downloadAllImages = async (urls) => {
const downloadPromises = urls.map((url, index) => {
const filename = `image_${index + 1}.jpg`;
const savePath = path.resolve(downloadFolder, filename);
return downloadImage(url, savePath);
});
return Promise.all(downloadPromises);
};
downloadAllImages(imageUrls)
.then(() => {
console.log('All images downloaded successfully!');
})
.catch(error => {
console.error('Failed to download images:', error);
});
The method is very similar to downloading a single image. We just need to prepare a new directory and make sure it exists to store the images.
Then, we'll run a new method called downloadAllImages
to make the URLs and download the image one by one.
Improvement Idea
You can use PromiseAll method from Javascript to download the image simultaneously or use the Coroutine method for Python. Feel free to try!