If you want to get Google Maps data, you can use the Google Maps API to retrieve the information you need. Alternatively, you can SERP API services like SerpApi - Google Maps API documentation. Our API allows you to scrape SERP results from Google Maps searches without any headache to manage document changes, bypass anti scraping protection, and proxies. In this article, I will demo how to retrieve the data you need in only a few simple steps using Javascript.
Playground
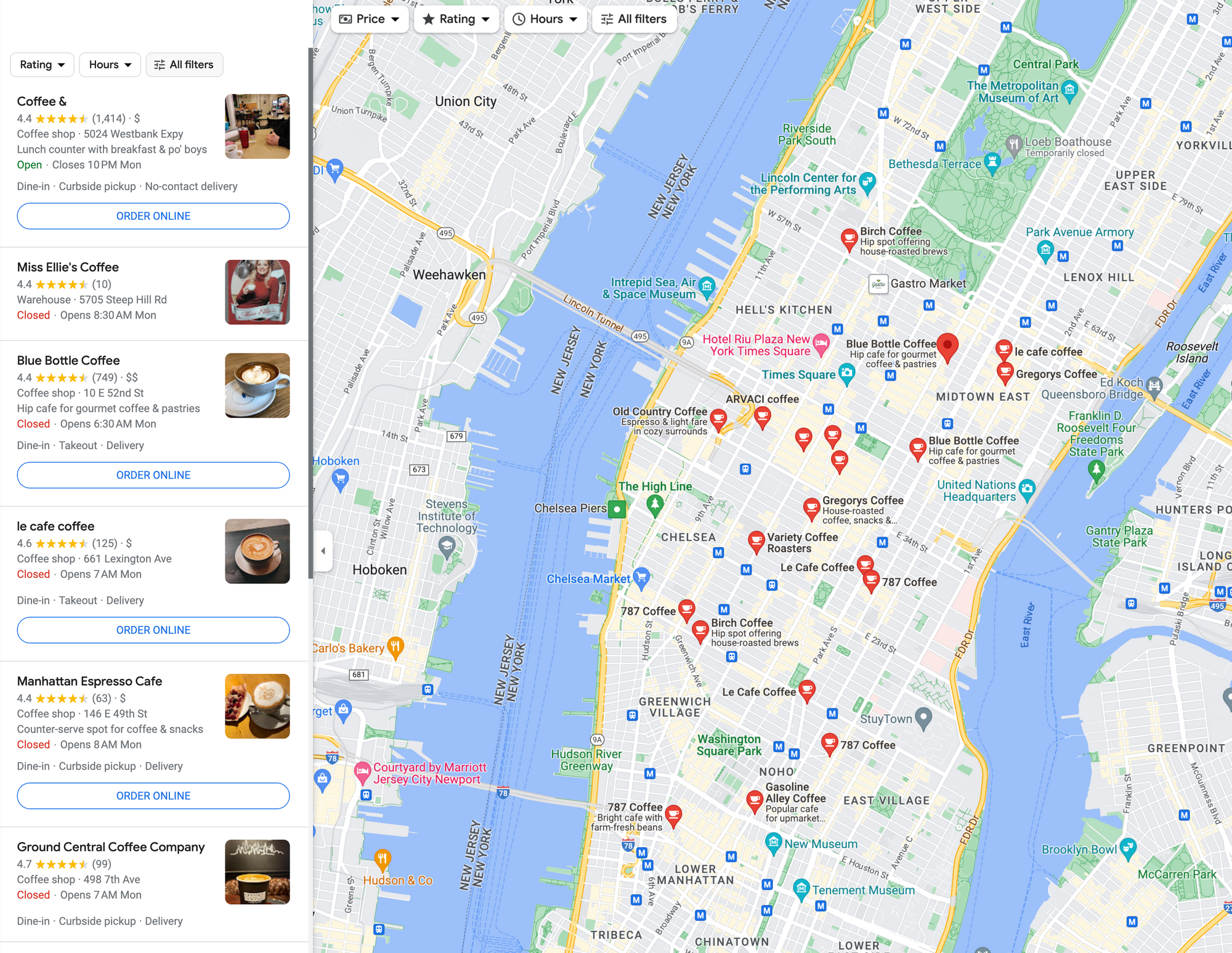
You can try it out in our playground for the above search (no sign up required). This is the actual data from the search using our API.
"local_results": [
{
"position": 1,
"title": "Coffee &",
"place_id": "ChIJf732s2-kIIYRfJH0710yoUU",
"data_id": "0x8620a46fb3f6bd7f:0x45a1325deff4917c",
"data_cid": "5017346838906573180",
"reviews_link": "https://serpapi.com/search.json?data_id=0x8620a46fb3f6bd7f%3A0x45a1325deff4917c&engine=google_maps_reviews&hl=en",
"photos_link": "https://serpapi.com/search.json?data_id=0x8620a46fb3f6bd7f%3A0x45a1325deff4917c&engine=google_maps_photos&hl=en",
"gps_coordinates": {
"latitude": 29.895196,
"longitude": -90.1011485
},
"place_id_search": "https://serpapi.com/search.json?engine=google_maps&google_domain=google.com&hl=en&place_id=ChIJf732s2-kIIYRfJH0710yoUU",
"rating": 4.4,
"reviews": 1414,
"price": "$",
"type": "Coffee shop",
"address": "5024 Westbank Expy, Marrero, LA 70072",
"open_state": "Open ⋅ Closes 10 PM Mon",
"hours": "Open ⋅ Closes 10 PM Mon",
"operating_hours": {
"sunday": "Open 24 hours",
"monday": "12 AM–10 PM",
"tuesday": "Open 24 hours",
"wednesday": "Open 24 hours",
"thursday": "Open 24 hours",
"friday": "Open 24 hours",
"saturday": "Open 24 hours"
},
"phone": "(504) 328-9494",
"website": "https://coffeeandmarrero.com/",
"description": "Lunch counter with breakfast & po' boys. Donuts & breakfast all day, plus Cajun lunch bites, all in a '70s-era diner with counter seating.",
"service_options": {
"dine_in": true,
"curbside_pickup": true,
"no_contact_delivery": true
},
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipMaNRM6n8PObw2w_06yuqtpEMhX2zKTYm1h68WR=w80-h106-k-no"
},
{
"position": 2,
"title": "Miss Ellie's Coffee",
"place_id": "ChIJiS41zBaty4cRSXHxtMCSpv4",
"data_id": "0x87cbad16cc352e89:0xfea692c0b4f17149",
"data_cid": "18349515088134762825",
"reviews_link": "https://serpapi.com/search.json?data_id=0x87cbad16cc352e89%3A0xfea692c0b4f17149&engine=google_maps_reviews&hl=en",
"photos_link": "https://serpapi.com/search.json?data_id=0x87cbad16cc352e89%3A0xfea692c0b4f17149&engine=google_maps_photos&hl=en",
"gps_coordinates": {
"latitude": 35.2832303,
"longitude": -94.37262199999999
},
"place_id_search": "https://serpapi.com/search.json?engine=google_maps&google_domain=google.com&hl=en&place_id=ChIJiS41zBaty4cRSXHxtMCSpv4",
"rating": 4.4,
"reviews": 10,
"unclaimed_listing": true,
"type": "Warehouse",
"address": "5705 Steep Hill Rd, Fort Smith, AR 72916",
"open_state": "Closed ⋅ Opens 8:30 AM Mon",
"hours": "Closed ⋅ Opens 8:30 AM Mon",
"operating_hours": {
"sunday": "Closed",
"monday": "8:30 AM–4:30 PM",
"tuesday": "8:30 AM–4:30 PM",
"wednesday": "8:30 AM–4:30 PM",
"thursday": "8:30 AM–4:30 PM",
"friday": "8:30 AM–4:30 PM",
"saturday": "Closed"
},
"phone": "(800) 344-2739",
"website": "http://www.coffee.org/",
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipOgK4NZjQ8hnCvWe_uqaM8OMP-rWFQBhw5KqN23=w80-h142-k-no"
},
...
]
The code
import { getJson } from "serpapi";
const API_KEY = process.env['API_KEY'] // Get your API_KEY from https://serpapi.com/manage-api-key
async function getReviews(dataId) {
const response = await getJson("google_maps_reviews", {
api_key: API_KEY,
data_id: dataId
})
return response["reviews"]
}
async function getPhotos(dataId) {
const response = await getJson("google_maps_photos", {
api_key: API_KEY,
data_id: dataId
})
return response["photos"]
}
async function getResults(query) {
const response = await getJson("google_maps", {
api_key: API_KEY,
q: query,
ll: "@40.7455096,-74.0083012,14z"
})
const localResults = response["local_results"]
for (let i = 0; i < localResults.length; i++) {
localResults[i]["reviews"] = await getReviews(localResults[i]["data_id"])
localResults[i]["photos"] = await getPhotos(localResults[i]["data_id"])
}
return localResults
}
console.log(await getResults("Coffee"))
Fork this code in Replit and play around with it. There are 3 important parts in the above. First we retrieve the search result using Google Maps API. Once we have the result, we can use data_id
provided in the response to retrieve the reviews
and photos
of each restaurant
.
Reviews API allow you to retrieve users' reviews of the restaurant or business, which consists of user profile
, rating
, date
, images
if any and etc. It can be useful if you want to analyse the overall customer satisfaction of the business. While Photos API allow you to retrieve user posted photos, thumbnail
and full size image
are available from the API.
Sample response from above code:
{
position: 1,
title: 'Blue Bottle Coffee',
place_id: 'ChIJfYfBk_tYwokRozvC5UCvOWM',
data_id: '0x89c258fb93c1877d:0x6339af40e5c23ba3',
data_cid: '7149938576694852515',
reviews_link: 'https://serpapi.com/search.json?data_id=0x89c258fb93c1877d%3A0x6339af40e5c23ba3&engine=google_maps_reviews&hl=en',
photos_link: 'https://serpapi.com/search.json?data_id=0x89c258fb93c1877d%3A0x6339af40e5c23ba3&engine=google_maps_photos&hl=en',
gps_coordinates: { latitude: 40.7596054, longitude: -73.97548789999999 },
place_id_search: 'https://serpapi.com/search.json?engine=google_maps&google_domain=google.com&hl=en&place_id=ChIJfYfBk_tYwokRozvC5UCvOWM',
rating: 4.4,
reviews: [
{
link: 'https://www.google.com/maps/reviews/data=!4m8!14m7!1m6!2m5!1sChdDSUhNMG9nS0VJQ0FnSUN1NW9yQzFRRRAB!2m1!1s0x0:0x6339af40e5c23ba3!3m1!1s2@1:CIHM0ogKEICAgICu5orC1QE%7CCgwIu9iElwYQuPaUzQE%7C?hl=en-US',
user: [Object],
rating: 5,
date: '9 months ago',
snippet: 'The cold brew with oat milk was really good. Smooth and not watery. I am not sure which oat milk they use but it was creamy. They do have a large seating table then some smaller tables on the back with one booth. They apparently only have one type of cup and lid for both iced and hot drinks. My issue is that the opening on the lids is not large enough for a straw. I like to use a straw for iced drinks. They have papers straws so you can squeeze them in. Just for 7 dollar iced coffee i would like at least a larger opening on the lid.',
likes: 2,
images: [{...}]
},
...
],
price: '$$',
type: 'Coffee shop',
address: '10 E 52nd St, New York, NY 10022',
open_state: 'Closed ⋅ Opens 6:30 AM Mon',
hours: 'Closed ⋅ Opens 6:30 AM Mon',
operating_hours: {
sunday: '8 AM–6 PM',
monday: '6:30 AM–6 PM',
tuesday: '6:30 AM–6 PM',
wednesday: '6:30 AM–6 PM',
thursday: '6:30 AM–6 PM',
friday: '6:30 AM–6 PM',
saturday: '8 AM–6 PM'
},
phone: '(510) 653-3394',
website: 'https://bluebottlecoffee.com/',
description: 'Hip cafe for gourmet coffee & pastries. Trendy cafe chain offering upscale coffee drinks & pastries, plus beans & brewing equipment.',
service_options: { dine_in: true, takeout: true, delivery: true },
thumbnail: 'https://lh5.googleusercontent.com/p/AF1QipOEYg1tmTlSwR5eMUy4jjbdWwJgMMUUM9wB1d5H=w92-h92-k-no',
photos: [
{
thumbnail: 'https://lh5.googleusercontent.com/p/AF1QipO8aNgnjMg1iYJ3yJ8v-SiTk1oXPv97cW91nhj5=w203-h152-k-no',
image: 'https://lh5.googleusercontent.com/p/AF1QipO8aNgnjMg1iYJ3yJ8v-SiTk1oXPv97cW91nhj5=w4032-h3024-k-no',
photo_meta_serpapi_link: 'https://serpapi.com/search.json?data_id=AF1QipO8aNgnjMg1iYJ3yJ8v-SiTk1oXPv97cW91nhj5&engine=google_maps_photo_meta'
},
...
]
}
Documentation
If you have any questions, please feel free to reach out to me.
Add a Feature Request💫 or a Bug🐞