Scraping Google Hotels for hotels data can be valuable to your business. These data can be used for
- Analysis: Data such as pricing, availability, ratings, and reviews could be useful for market analysis, competitive research, and understanding pricing trends.
- Price Comparison: Compare prices across various platforms or to monitor hotel price changes over time. This can be particularly useful for travel agencies or price comparison websites.
- Feature Extraction: Gather data such as amenities, location, room types, and more.
- Sentiment Analysis: One can conduct sentiment analysis to gauge customer satisfaction and reputation of hotels from the users’ reviews.
- Machine Learning Model Training: Train machine learning models for various purposes like predictive pricing, recommendation systems, or to understand customer preferences.
In SerpApi, we provide easy and fast API to scrape various search engines like Google, Google Maps, Bing, other engine that under Google Travel like Google Flights and many others. In this blog post, we will be using Google Hotels API to scrape Google Hotels.
If you are looking for a complete step-by-step tutorial to build your own Hotels data scraper, we have blog post that cover it. It includes scraping data from booking.com, Airbnb and etc.
If you are wondering the legality of web scraping, you can checkout our FAQ. Scraping data that is publicly available is generally safe provided the usage is fully legal.
Playground
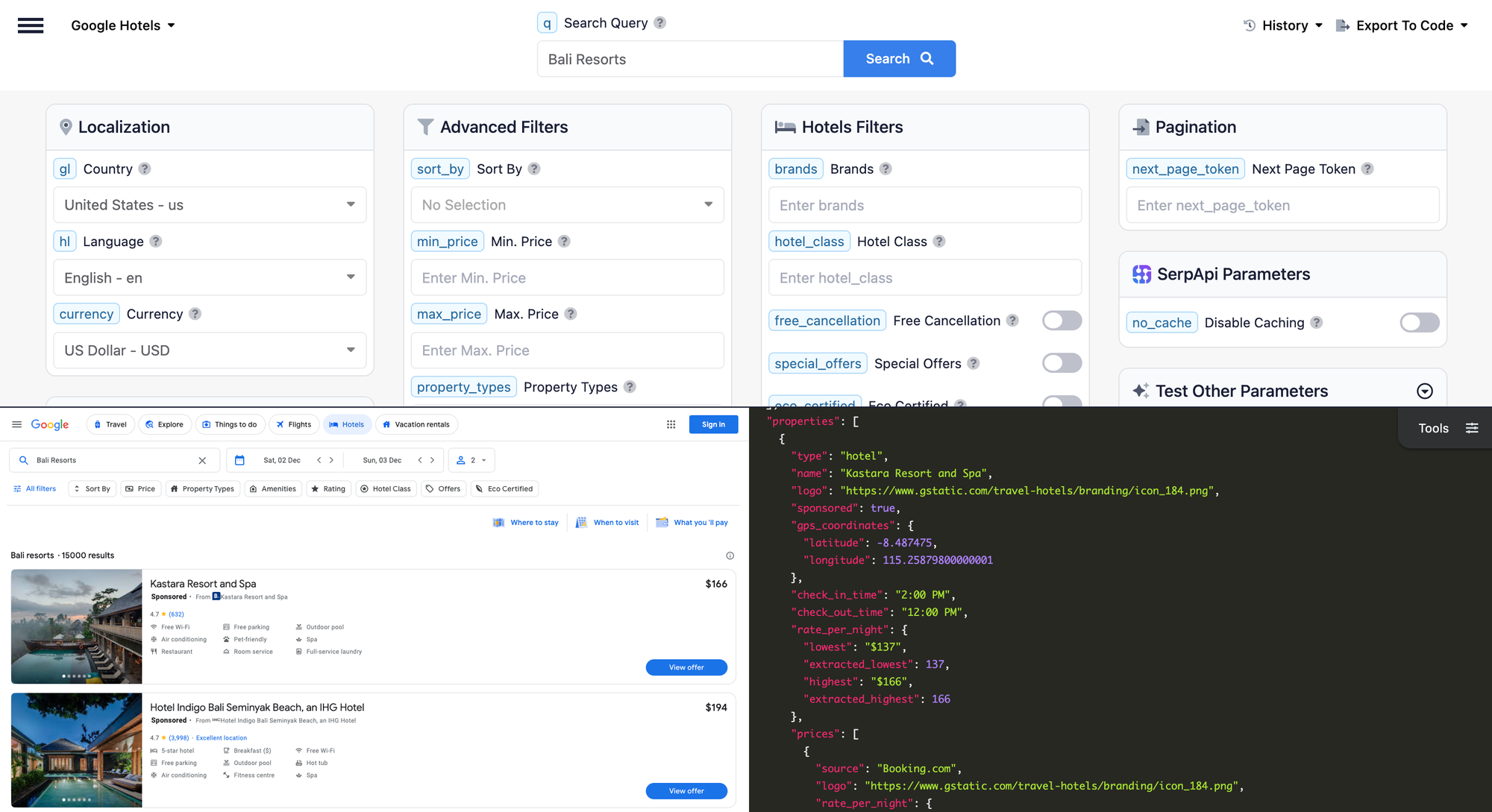
We have a playground for the API that you can test out. Playground allow you to visualise the data that you can get from our API and compare it with the data shown in Google Hotels itself.
Why use an API?
- No need to create a parser from scratch and maintain it.
- Bypass blocks from Google: solve CAPTCHA or solve IP blocks.
- No need to pay for proxies, and CAPTCHA solvers.
- Don't need to use browser automation.
SerpApi takes care of everything mentioned above with fast response times under ~2.47 seconds
(~1.33 seconds
with Ludicrous speed) per request. The result is a well structured data in JSON with only a single API call.
Response times and success rates are shown under the SerpApi Status page.
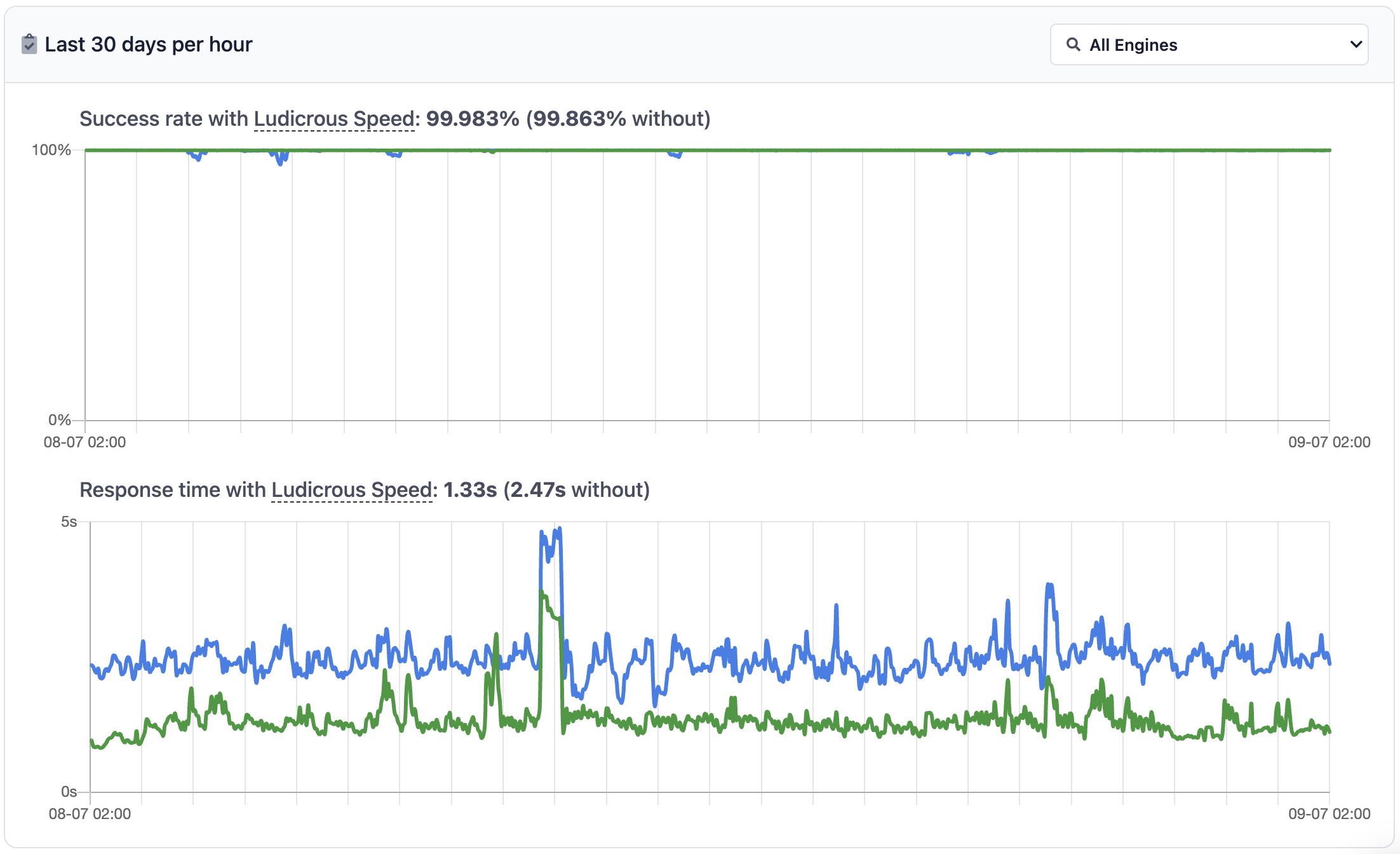
Full code in Javascript
If you don't need an explanation, feel free to copy and paste the code into your favorite IDE and start running. Otherwise, you can follow the step-by-step code explanation to know what each line is doing. You can also check out our playground, which is very handy to play around with the API and get a sense of how things work.
import { getJson } from "serpapi"
const SERPAPI_KEY = "..." // Get your API_KEY from https://serpapi.com/manage-api-key
const googleHotelsParams = {
api_key: SERPAPI_KEY,
engine: "google_hotels",
q: "Bali Resorts",
check_in_date: "2024-01-20",
check_out_date: "2024-01-25",
adults: 2,
}
const googleHotelsResponse = await getJson(googleHotelsParams)
Prerequisite
Install library:
npm install serpapi
serpapi is an official SerpApi API package. Follow this guide to get your Node.js and npm
install if you haven’t done so.
Code Explanation
First, we need to import the library
import { getJson } from "serpapi"
We set the required parameters for Google Hotels API. A list of parameters and their detailed explanation can be found on our documentation. q
is hotel search query that can be anything available in Google Hotels like Iceland, New York. For this demo, we are scraping the Bali Resorts
hotels data.
const googleHotelsParams = {
api_key: SERPAPI_KEY,
engine: "google_hotels",
q: "Bali Resorts",
check_in_date: "2024-01-20",
check_out_date: "2024-01-25",
adults: 2,
}
Once parameters are defined, everything else will be taken care of by the library, for example, making the actual API request. Calling getJson
to make the API request and JSON data will be returned and assigned it to googleHotelsResponse
.
const googleHotelsResponse = await getJson(googleHotelsParams)
Output:
The total number of results per page is about 20
. The data includes hotel name, price data, customer reviews, amenities and other hotel details.
console.log(googleHotelsResponse)
"properties": [
{
"type": "hotel",
"name": "Sol Benoa Bali - All Inclusive",
"description": "Chic hotel opposite a private area of beach, plus dining, bars, a spa & an outdoor pool.",
"logo": "https://www.gstatic.com/travel-hotels/branding/icon_49.png",
"sponsored": true,
"gps_coordinates": {
"latitude": -8.78685,
"longitude": 115.22636500000002
},
"check_in_time": "3:00 PM",
"check_out_time": "12:00 PM",
"rate_per_night": {
"lowest": "$103",
"extracted_lowest": 103,
"highest": "$124",
"extracted_highest": 124
},
"prices": [
{
"source": "Sol Benoa Bali - All Inclusive",
"logo": "https://www.gstatic.com/travel-hotels/branding/icon_49.png",
"rate_per_night": {
"lowest": "$103",
"extracted_lowest": 103,
"highest": "$124",
"extracted_highest": 124
}
}
],
"nearby_places": [
{
"name": "Nusa Dua 2",
"transportations": [
{
"type": "Walking",
"duration": "9 min"
}
]
},
{
"name": "I Gusti Ngurah Rai International Airport",
"transportations": [
{
"type": "Taxi",
"duration": "18 min"
},
{
"type": "Public transport",
"duration": "30 min"
}
]
},
{
"name": "九九港式海鲜火锅 nine resturant",
"transportations": [
{
"type": "Walking",
"duration": "2 min"
}
]
}
],
"hotel_class": "5-star hotel",
"extracted_hotel_class": 5,
"images": [
{
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipM2c6Pq3LHe0_vVOawTPwExOyVgljaM6XtRWbYB=s287-w287-h192-n-k-no-v1",
"original_image": "https://lh5.googleusercontent.com/p/AF1QipM2c6Pq3LHe0_vVOawTPwExOyVgljaM6XtRWbYB=s10000"
},
{
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipNvK84tnuQFSmrXSkmksZ7cPDh4pnCCTEZ6jXPN=s287-w287-h192-n-k-no-v1",
"original_image": "https://lh5.googleusercontent.com/p/AF1QipNvK84tnuQFSmrXSkmksZ7cPDh4pnCCTEZ6jXPN=s10000"
},
{
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipN18zikmhexJi89EzcJsvjSjYZx6UhK9V1xu5jf=s287-w287-h192-n-k-no-v1",
"original_image": "https://lh5.googleusercontent.com/p/AF1QipN18zikmhexJi89EzcJsvjSjYZx6UhK9V1xu5jf=s10000"
},
{
"thumbnail": "https://lh5.googleusercontent.com/proxy/A5Bhc2zyxpRWaKo-Mw9PZul02PY_CjLufpShbXQGKXlElDyaRwFK84aX7tlya-X8X0PmH1qCtTbedKkh25p3ooKRY5CzCj-bNOjolii3HPXK0tJsGdOHBfCKS39z-bCbEY2dlyb81BfHUImxJacEPbNiG76e6Q=s287-w287-h192-n-k-no-v1",
"original_image": "https://images.trvl-media.com/lodging/1000000/70000/69000/68930/cc9846c6_z.jpg"
},
{
"thumbnail": "https://lh4.googleusercontent.com/proxy/59RwMT-xvuxsNOQXgr-tnkLvojkuCD4dIpxfsKo6-B701ajAyuJRGxIkn82NzABtYS1WdbYiVSrmwElRuuzyBA80jagH4BIovclvHG3HLssHh9pmkSM5z34vHKa1Q4q0USrSHem0deWn-PGMPRGHmByemriaTA=s287-w287-h192-n-k-no-v1",
"original_image": "https://photos.hotelbeds.com/giata/original/02/024222/024222a_hb_ro_021.jpg"
},
{
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipM7OJlT7axQs7KgpCmeTMoAYaZ8aEDKSxUhqRGe=s287-w287-h192-n-k-no-v1",
"original_image": "https://lh5.googleusercontent.com/p/AF1QipM7OJlT7axQs7KgpCmeTMoAYaZ8aEDKSxUhqRGe=s10000"
},
{
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipOgxmRnvCX6liordKlrAhhsLG4vbMHjgNaMGF1W=s287-w287-h192-n-k-no-v1",
"original_image": "https://lh5.googleusercontent.com/p/AF1QipOgxmRnvCX6liordKlrAhhsLG4vbMHjgNaMGF1W=s10000"
},
{
"thumbnail": "https://lh4.googleusercontent.com/proxy/qnya5wZNzzsF0jqbJrPUpdxGRsLCkgmwi5sabFoM2lRaFNdnH7LIwdKYSFWgDZuLqLDO2qnvtgywNkSgUmPlHcbygmUxr9TGj9OXbnbSP1LQGIGZ-a6q-_ZntDk3bdCSVPlK4ITNtm4FQA7aK44nHZ2wUQkujw=s287-w287-h192-n-k-no-v1",
"original_image": "https://photos.hotelbeds.com/giata/original/02/024222/024222a_hb_p_024.jpg"
},
{
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipOkZYPJDtT0E8L_VZUYtj5tW3eTgMekluTkSXsp=s287-w287-h192-n-k-no-v1",
"original_image": "https://lh5.googleusercontent.com/p/AF1QipOkZYPJDtT0E8L_VZUYtj5tW3eTgMekluTkSXsp=s10000"
}
],
"overall_rating": 4.5,
"reviews": 1288,
"location_rating": 3.4,
"reviews_breakdown": [
{
"name": "Nature",
"description": "Nature and outdoor activities",
"total_mentioned": 173,
"positive": 127,
"negative": 23,
"neutral": 23
},
{
"name": "Service",
"description": "Service",
"total_mentioned": 217,
"positive": 181,
"negative": 22,
"neutral": 14
},
{
"name": "Property",
"description": "Property",
"total_mentioned": 272,
"positive": 214,
"negative": 37,
"neutral": 21
},
{
"name": "Dining",
"description": "Food and Beverage",
"total_mentioned": 104,
"positive": 71,
"negative": 26,
"neutral": 7
},
{
"name": "Fitness",
"description": "Fitness",
"total_mentioned": 100,
"positive": 70,
"negative": 14,
"neutral": 16
},
{
"name": "Bar",
"description": "Bar or lounge",
"total_mentioned": 56,
"positive": 38,
"negative": 12,
"neutral": 6
}
],
"amenities": [
"Breakfast ($)",
"Free Wi-Fi",
"Free parking",
"Outdoor pool",
"Air conditioning",
"Fitness centre",
"Spa",
"Beach access",
"Bar",
"Restaurant",
"Room service",
"Full-service laundry",
"Accessible",
"Business centre",
"Child-friendly",
"Smoke-free property"
]
},
...
]
Pagination
When there is next page results, our API will return serpapi_pagination
. The next_page_token
can be used to retrieve the next page results.
{
"current_from": 1,
"current_to": 20,
"next_page_token": "CBI=",
"next": "https://serpapi.com/search.json?adults=2&check_in_date=2024-01-20&check_out_date=2024-01-25&children=0¤cy=USD&engine=google_hotels&gl=us&hl=en&next_page_token=CBI%3D&q=Bali+Resorts"
}
For example:
import { getJson } from "serpapi"
const SERPAPI_KEY = "..." // Get your API_KEY from https://serpapi.com/manage-api-key
const googleHotelsParams = {
api_key: SERPAPI_KEY,
engine: "google_hotels",
q: "Bali Resorts",
check_in_date: "2024-01-20",
check_out_date: "2024-01-25",
adults: 2,
next_page_token: "CBI="
}
const googleHotelsResponse = await getJson(googleHotelsParams)
Documentation
Conclusions
Scraping Google Hotels data can be valuable in terms of pricing strategy, pricing trends, market research, sentiment analysis, predictive analysis and etc. In future, we will be covering more in-depth hotel data, follow our public roadmap to get up to date information. In SerpApi, we provide an easy and fast API for our customers so they can focus the resources on their core of the business.
If you have any questions, please feel free to reach out to me.
Add a Feature Request💫 or a Bug🐞