The SerpApi Google Hotels API is a user-friendly solution for developers who want to integrate real-time hotel pricing data into their applications. It provides access to essential information such as rates, check-in/out times, and amenities without the hassle of web scraping. Whether you’re creating a comparison tool or enhancing a travel app, this API streamlines the entire process.
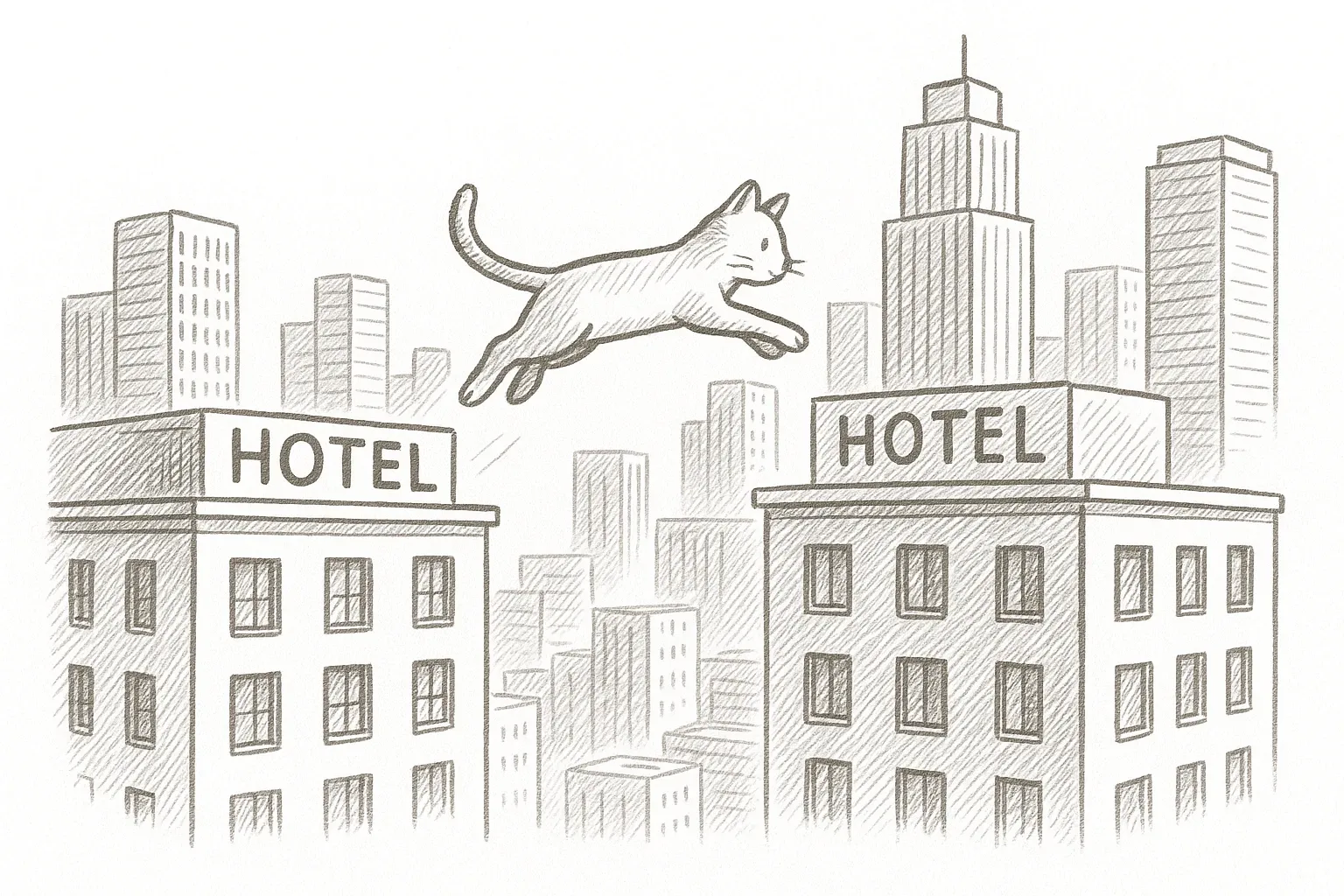
Google Hotels API
Here is the data example you can expect when using SerpApi's Google Hotels API.
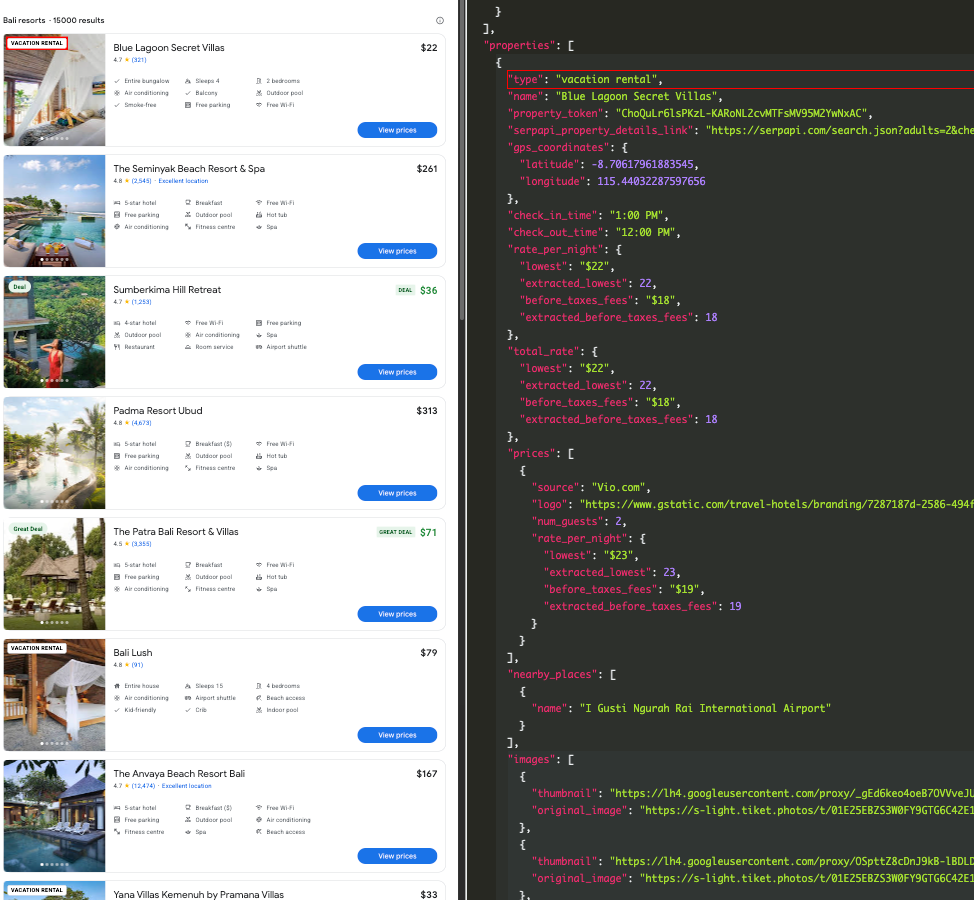
As you can see, not just prices information, you can see other information like amenities, rating, reviews, and more.
We also provide Google Hotels Property Details API to scrape more data about individual hotels.
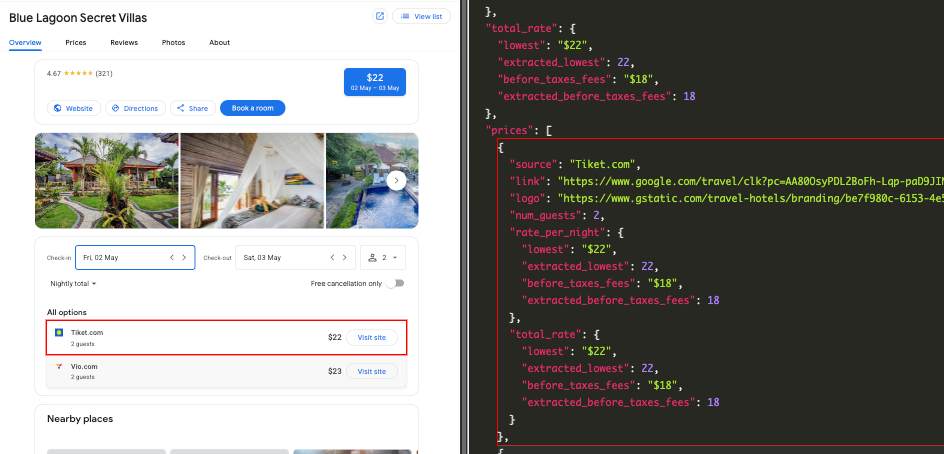
In case there are more options to book the hotel, we'll return all the sources and prices options through this API.
Code preparation
You can use a simple GET request or a dedicated SDK based on your programming language, including Python, JavaScript, and more. In this post, we'll use the GET request method in Python.
Preparation for accessing the SerpApi API
- Register at serpapi.com for free to get your SerpApi Api Key.
- Create a new
main.py
file - Install requests with
pip install requests
Here is what the basic setup looks like
import requests
SERPAPI_API_KEY = "YOUR_REAL_SERPAPI_API_KEY"
params = {
"api_key": SERPAPI_API_KEY, #replace with your real API Key
# soon
}
search = requests.get("https://serpapi.com/search", params=params)
response = search.json()
print(response)
With these few lines of code, we can access all of the search engines available at SerpApi, including Google Hotels API. We'll soon adjust the parameters based on our needs.
Scrape hotel prices
When searching for a hotel directly through the Google Travel site, we can see the prices listed on the right side like this:
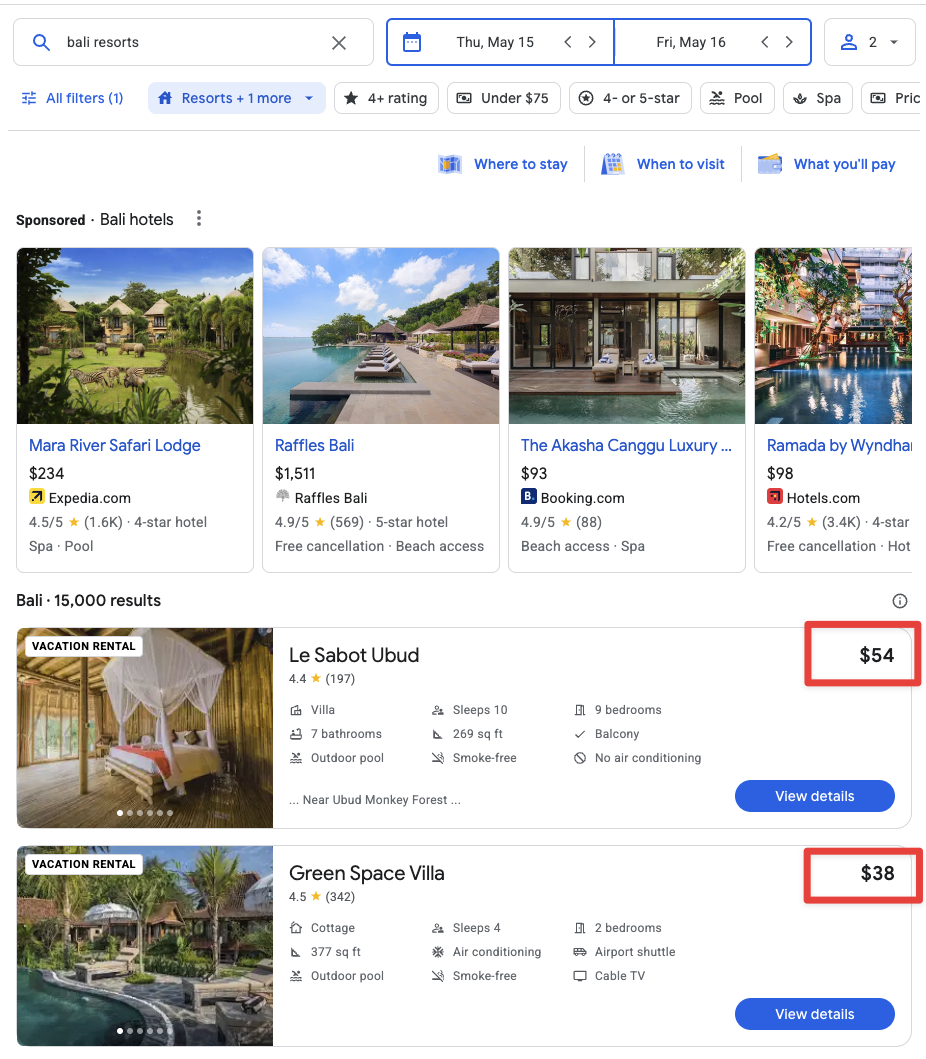
Let's learn how to scrape those prices.
We'll update our Python code to include the query we want to search for (in q
parameter) and the check-in and check-out dates in YYYY-MM-DD format.
import requests
SERPAPI_API_KEY = "YOUR_SERPAPI_API_KEY"
params = {
"api_key": SERPAPI_API_KEY,
"engine": "google_hotels",
"q": "Singapore",
"check_in_date": "2025-05-10",
"check_out_date": "2025-05-20",
}
search = requests.get("https://serpapi.com/search", params=params)
response = search.json()
print(response)
To make the output nicer, let's use the JSON package
import json
# ... all previous code
print(json.dumps(response, indent=2))
Now, under the properties
key, we can see the list of hotels, like this
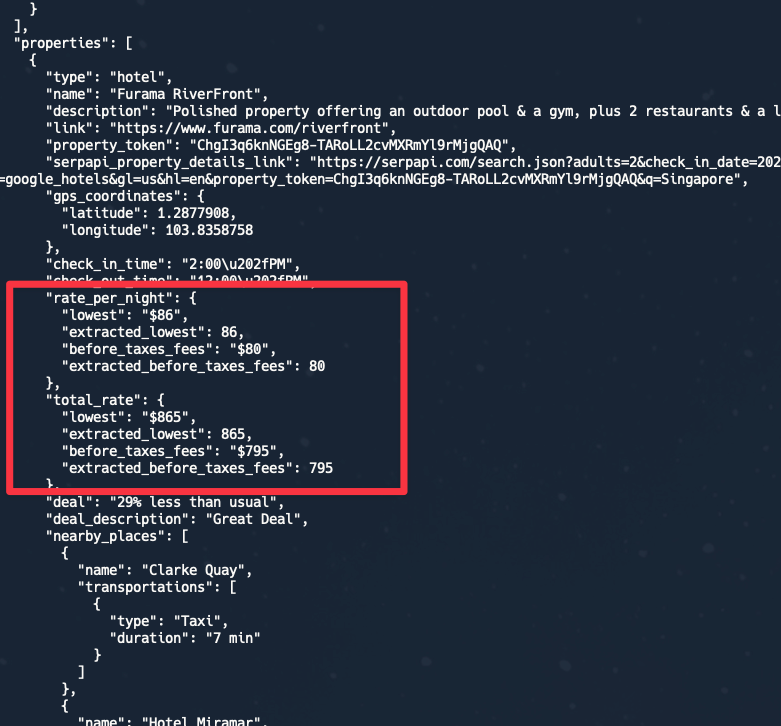
The rate_per_night
and total_rate
are available here.
Scrape more booking options
Additionally, when you click on one of the options on the Google Hotels site, we can see more prices listed on the individual page.
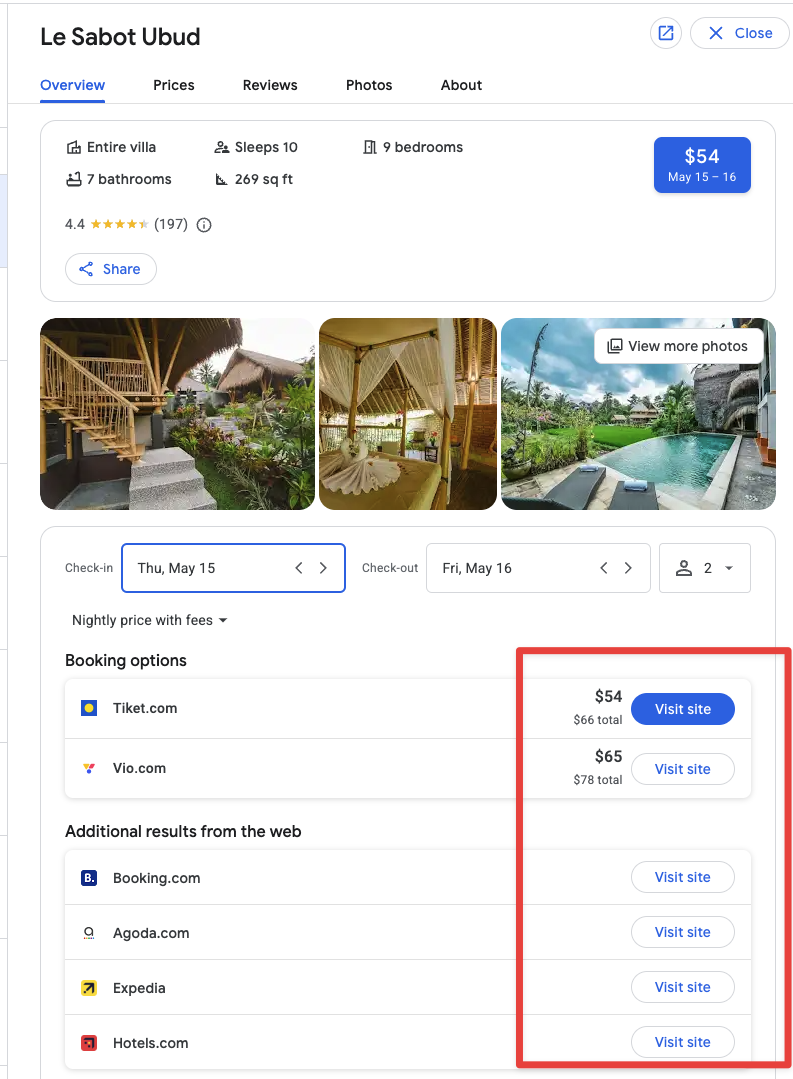
Let's learn how to scrape more prices or booking options like above.
Google Hotels Property Details API
In order to get the individual page information, we'll perform another request that requires a property_token
. This token is available upon initial request. The values are unique for each hotel. For example:
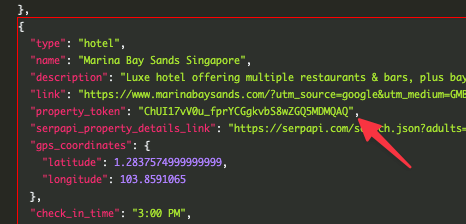
Reference: https://serpapi.com/google-hotels-property-details
Here is the adjusted code
import requests
import json
SERPAPI_API_KEY = "YOUR_SERPAPI_KEY" #replace with your real API Key
params = {
"api_key": SERPAPI_API_KEY,
"engine": "google_hotels",
"q": "Singapore",
"check_in_date": "2025-05-10",
"check_out_date": "2025-05-20",
"property_token": "ChUI17vV0u_fprYCGgkvbS8wZGQ5MDMQAQ",
}
search = requests.get("https://serpapi.com/search", params=params)
response = search.json()
print(json.dumps(response, indent=2))
I included the property_token manually; feel free to create a programmatic way if needed.
Now, you can see the list of prices in two different places:
- featured_prices
- prices
For the sponsored or featured prices, they're listed here:
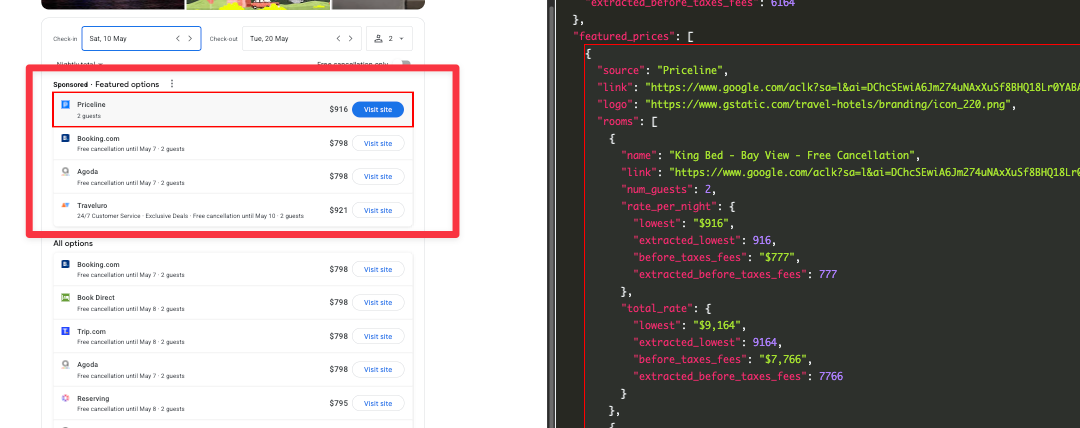
Next, for the regular prices:
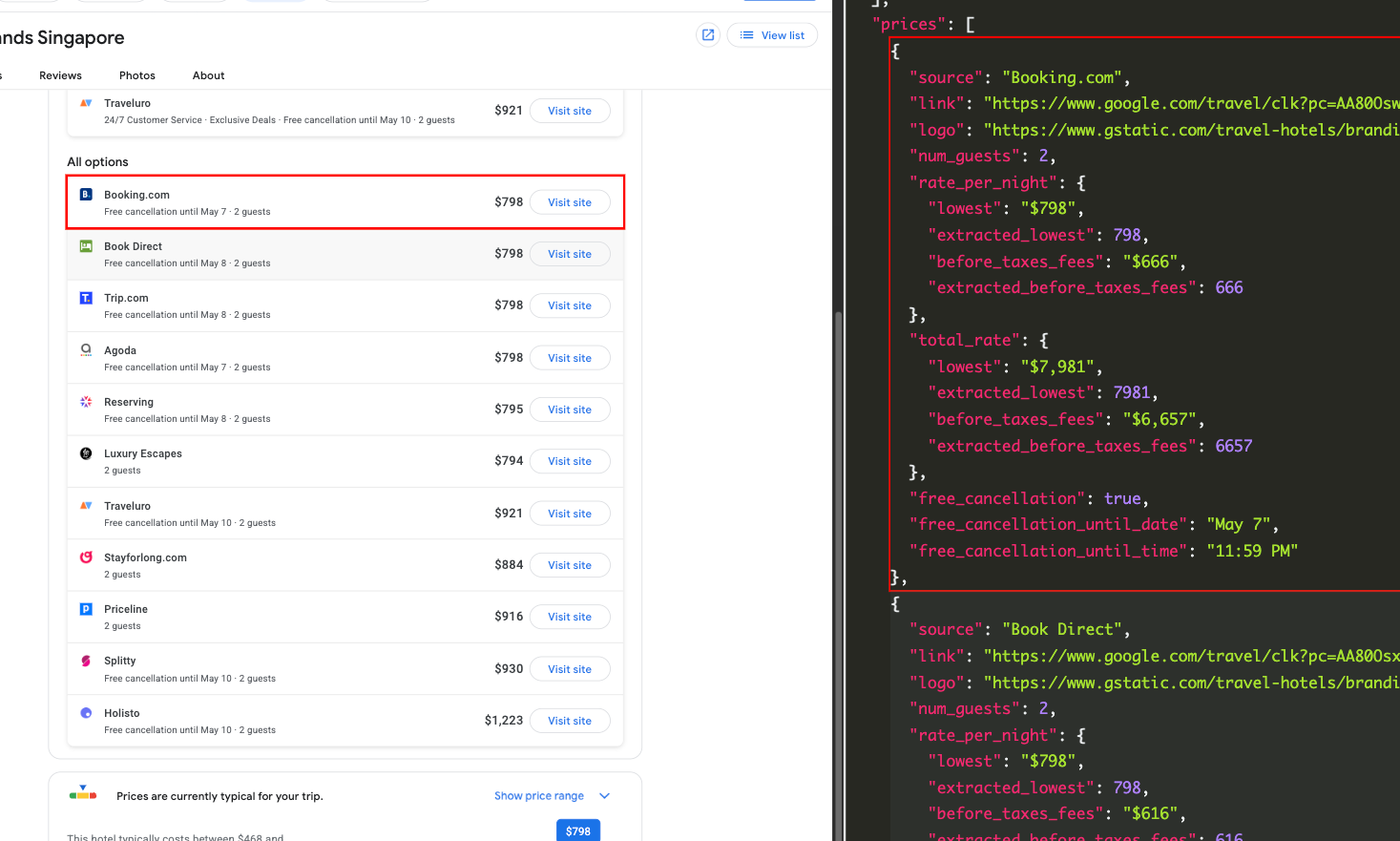
Depending on your use case, you might need this additional information.
More parameters
To refine your search, we provide additional parameters like gl, hl, currency, and ability to add filters like providing how many adults or children staying, as well as a way to sort the results.
You can take a look at all the available parameters here: https://serpapi.com/google-hotels-api
That's it, I hope you enjoy reading this article!
If you're interested in scraping the results in JavaScript, here is the related blog post:
We also have an interesting tutorial on how to track the hotel price using no-code tool:n8n