In my recent post announcing the Make app, I provided an example chaining SerpApi queries together to get additional data. For example, the Google Jobs API returns an array of jobs where each job has a job_id
and other data. You can then use the job_id
to look up more information by making a subsequent call to the Google Jobs Listing API.
The example I provided only ran the Google Jobs Listing lookup for the first job in the array. That's a helpful start, but what do you do if you want to iterate over the array of results and make a separate lookup for each element?
I'll explain how to do exactly that in this post. We'll use our Google Maps and Google Maps Reviews APIs to demonstrate this behavior. However, the steps for iterating over arrays and performing a subsequent action are the same regardless of which API or Module you're using.
For the sake of this example, we'll imagine we're running a taqueria trying to survive the fierce taco market of San Diego. We want to understand the competition and what customers think of each. In order to do this, we'll need to retrieve a lot of reviews. Fortunately, we can build this easily with SerpApi and Make.
Achieving this use case requires the following steps:
- Find taquerias in San Diego using the Google Maps API
- Fetch reviews for each taqueria using the Google Maps Reviews API
- Put the data somewhere like a CSV or Google Sheets
Let's go.
Scrape Google Maps with Make
Our first order of business is to find taquerias in the San Diego area via Google Maps. We'll do this by creating a new Scenario in Make and adding the SerpApi Search Google Maps Module to it.
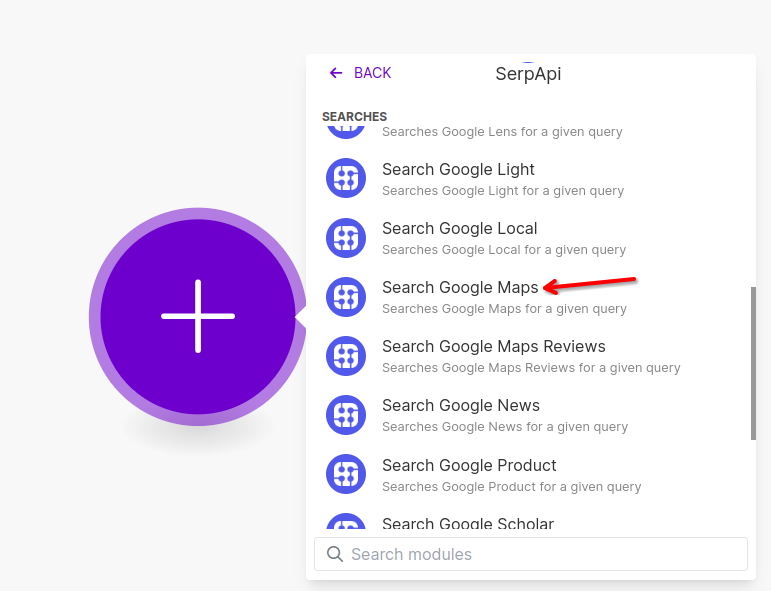
Make sure the SerpApi Module has a connection to your SerpApi account. If you don't see a Connection listed, follow the steps to create a connection with your SerpApi API key.
As for the "Search Query", you can simply put "taquerias near san diego, ca".
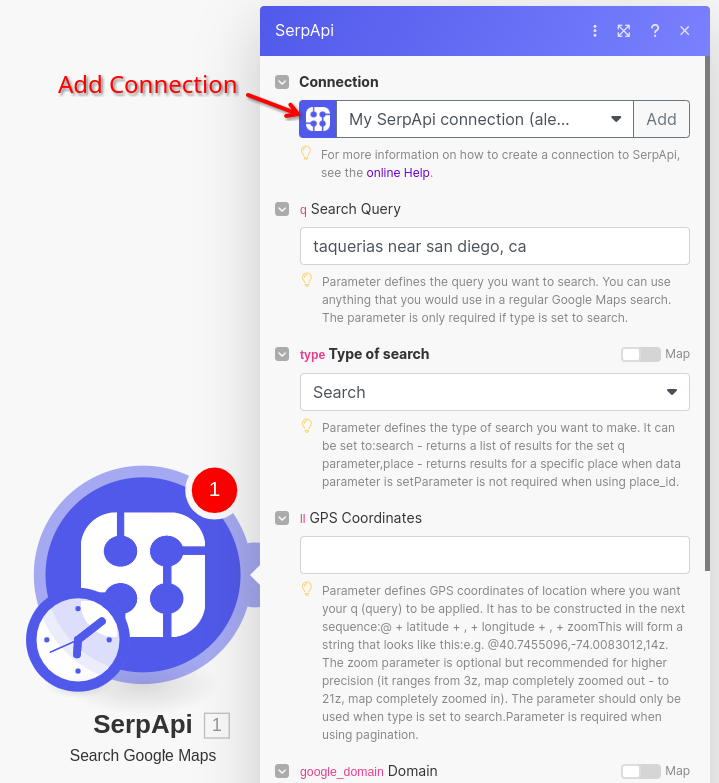
If you want to be more precise, you can also use "GPS Coordinates" to narrow the search area to San Diego only. We'll use @32.7002476,-117.1994717,12z
for San Diego.
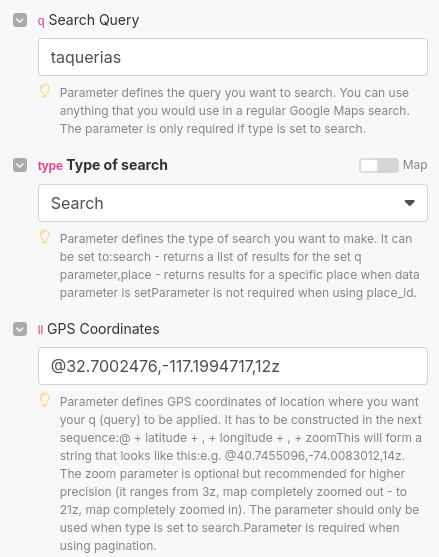
We hit the "Run once" button and should get some taqueria results in the "Local Results" array.
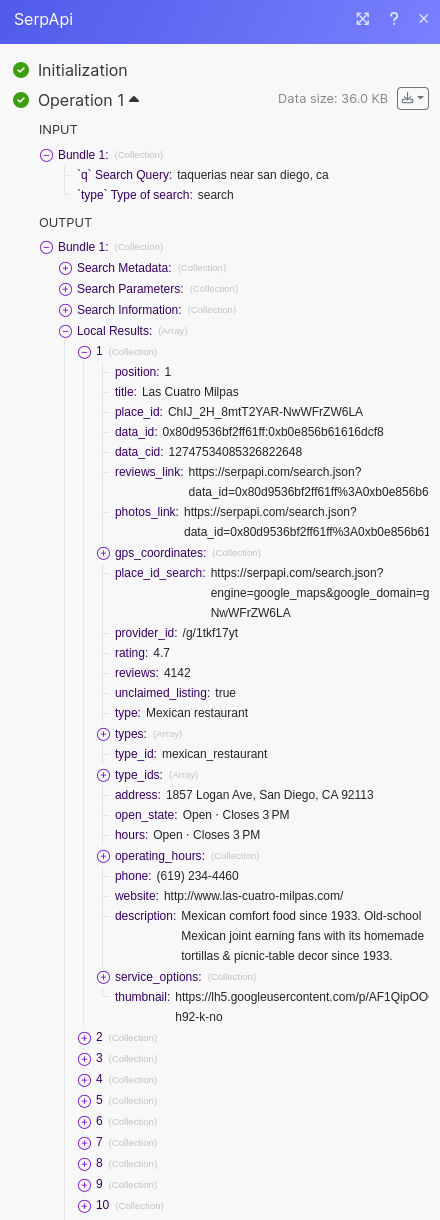
Now let's see how to iterate over that array.
Iterate over Google Maps Results
Find the "Flow Control" App which is a built-in collection of Modules created by Make. Add the "Iterator" Module to the Scenario.
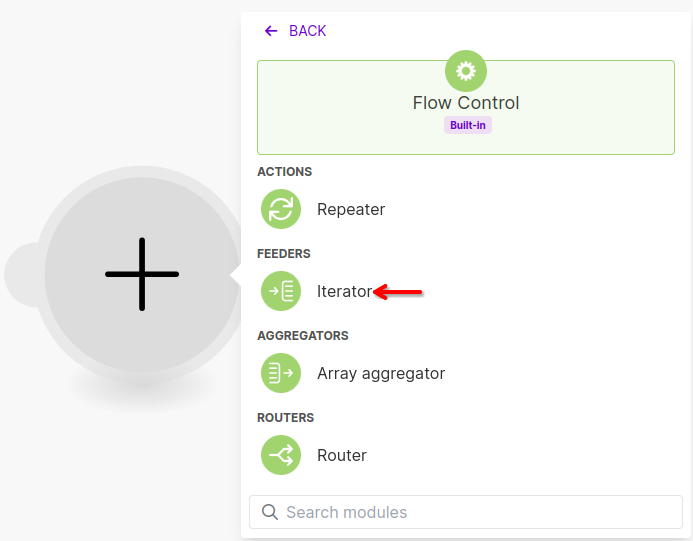
Once added, click within the "Array" form box to display the output fields of the Google Maps Search.
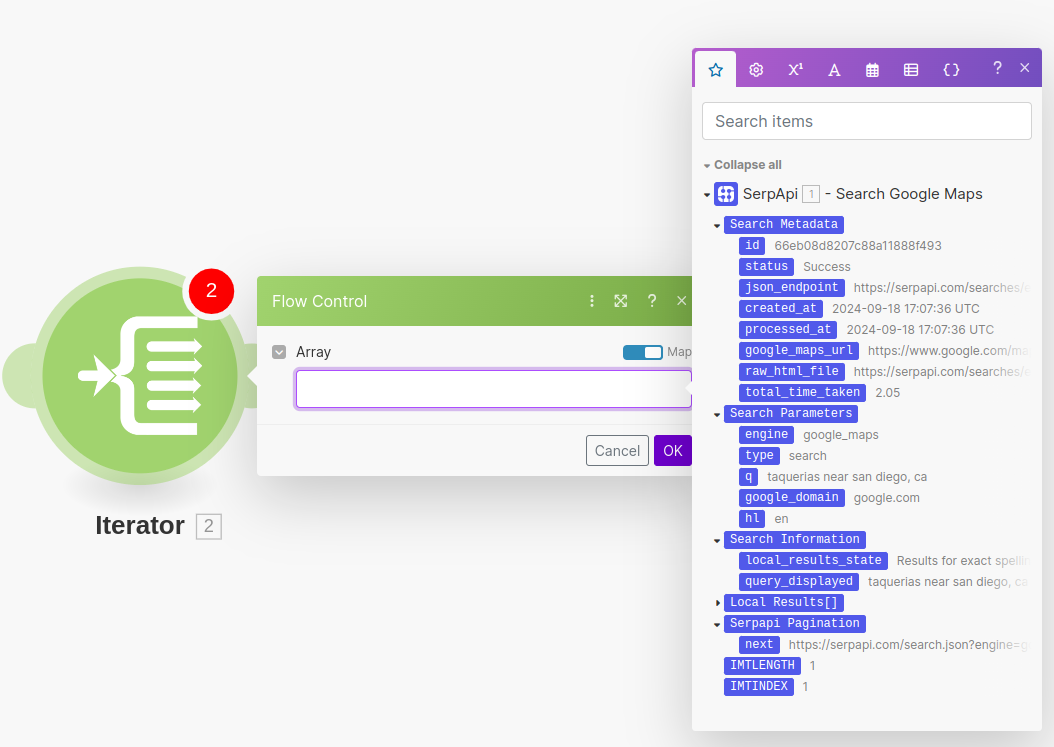
Click where it says Local Results[]
to set that as the array to iterate over. You should see that populate the "Array" form box.
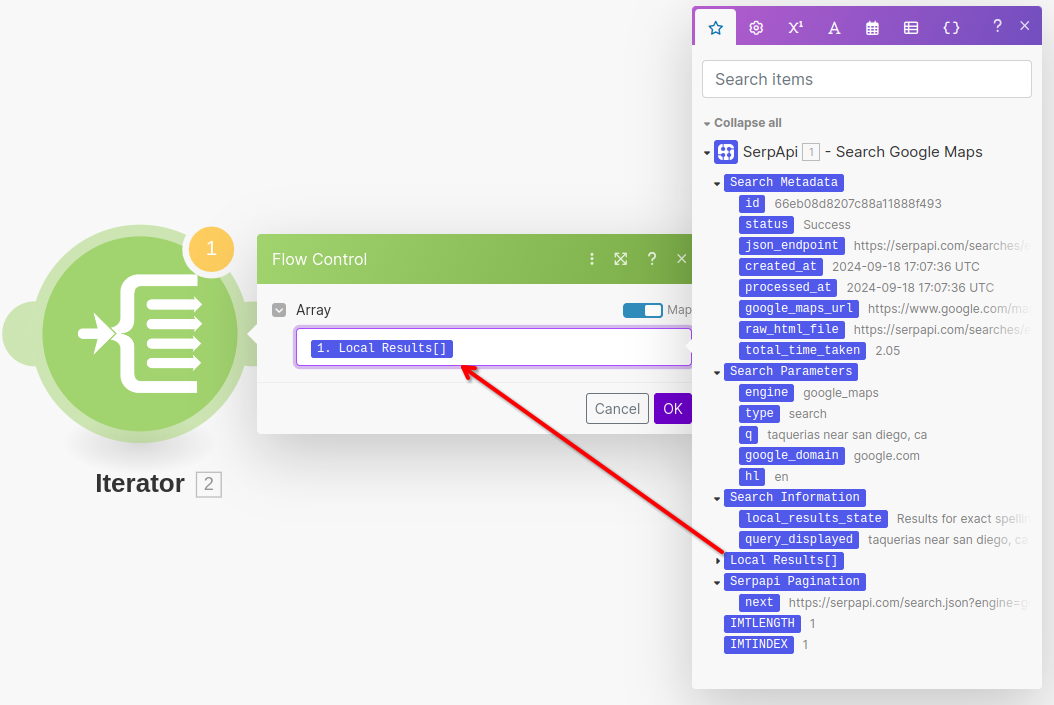
Unfortunately, we can't test this at this point because Make doesn't allow a "transformer" like the Iterator Module to be the last Module in a Scenario. Basically the Iterator needs a place to send each element of the iterated array before we know it's working. We'll do that by querying Google Maps Reviews.
Give this a test by clicking "Run once." You'll get a warning from Make, but you can ignore it and hit "Run anyway".
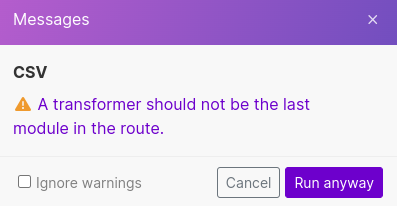
The Iterator should output a bundle for each taqueria.
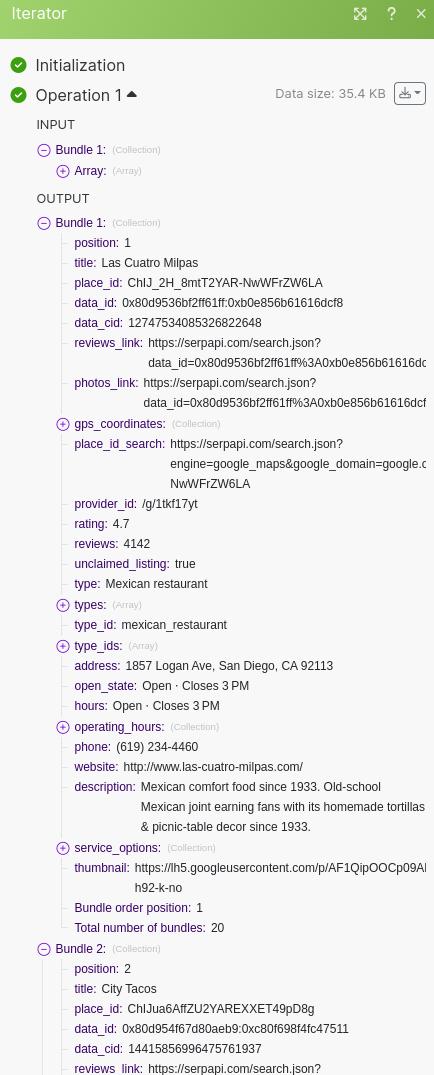
Scrape Google Maps Reviews with Make
We'll get our review data by adding the Search Google Maps Reviews Module.
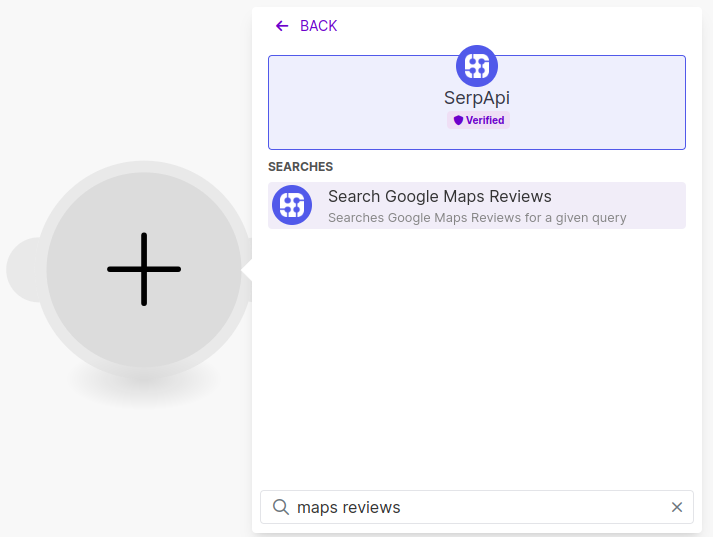
Click within the "Data ID" form box to display the output options of the Iterator Module. Click on data_id
to configure our Google Maps Reviews Module to search by this field. The form box should contain 2. data_id
. The 2
indicates the Module ID within the Scenario. In this case, it's the Iterator.
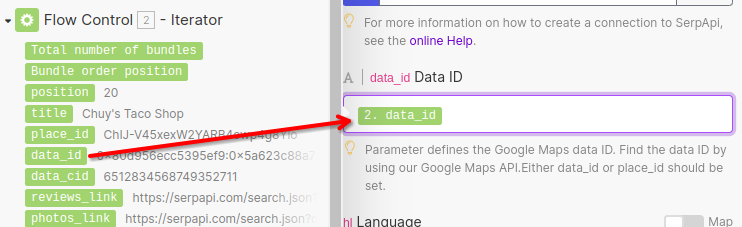
Hit the "Run once" button to give it a go. If all goes well, you should see the Scenario running for several seconds as it iterates over the Google Maps results and makes a subsequent query to Google Maps Reviews for each taqueria we found.
Here's what the Scenario should look like after completion. Notice the bubble coming off the Google Maps Reviews Module is "20" because it fetched reviews for 20 taquerias.
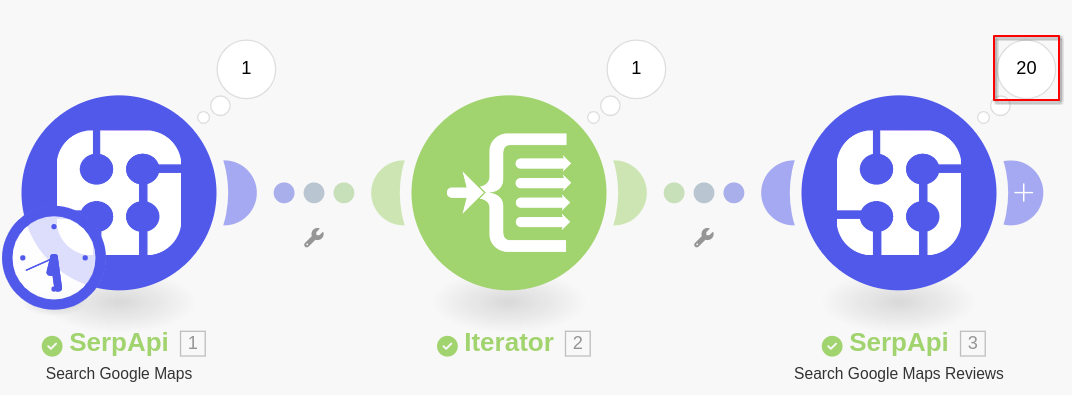
If you click on the "20", you can see the output of each Operation containing reviews for a single taqueria.
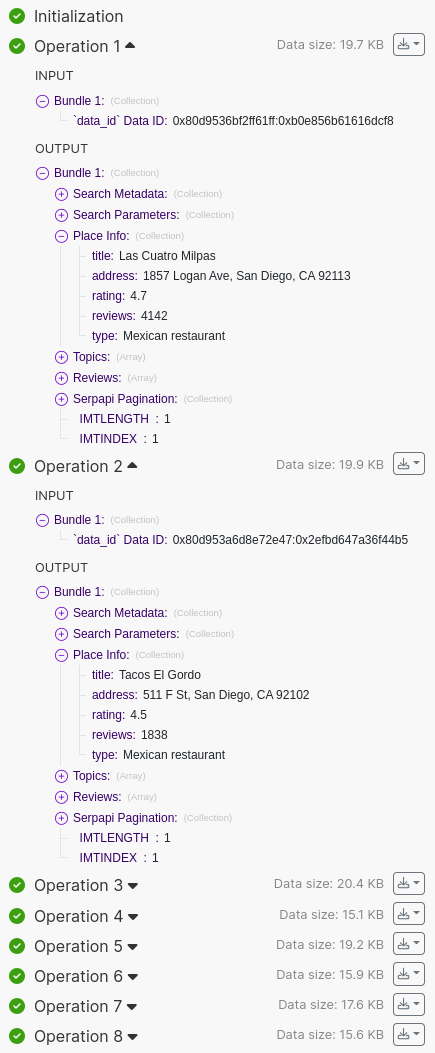
The reviews data we've been looking for is also an array so we'll actually need yet another Iterator to populate a CSV or Google Sheet with the review data.
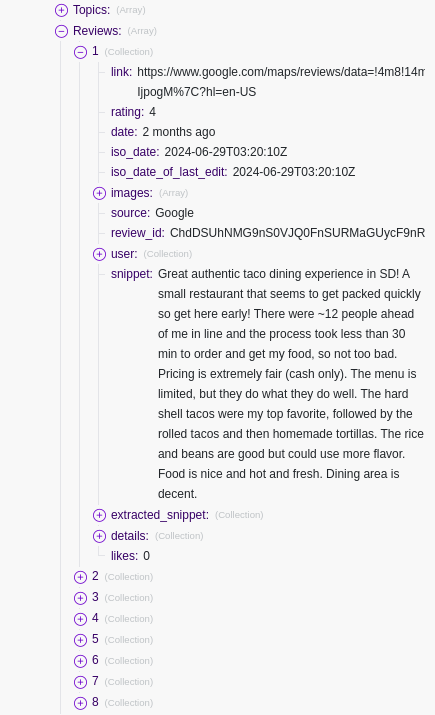
Iterate over Google Maps Reviews Results
This step is easy as it is basically identical to the previous Iterator we configured. This time we want to populate it with Reviews[]
.
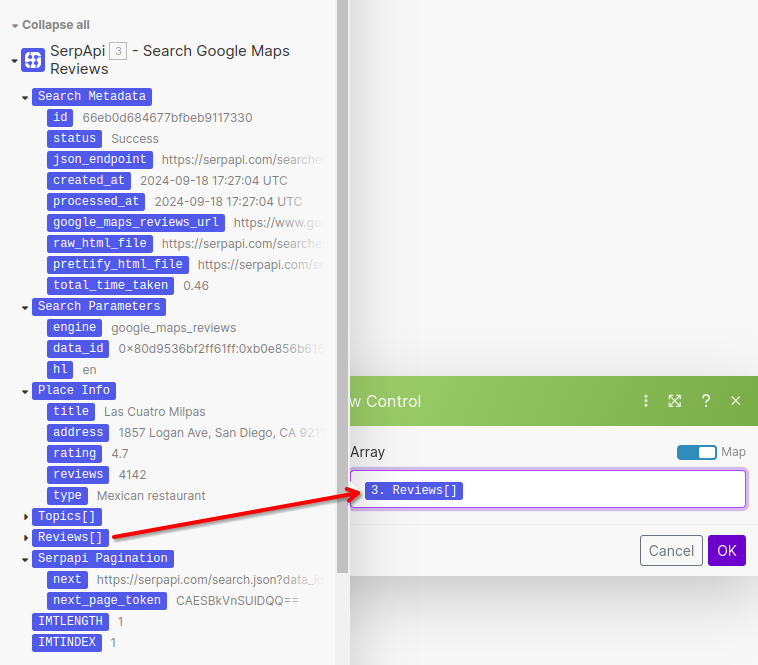
Run the Scenario once and you should get a collection of reviews for each taqueria.
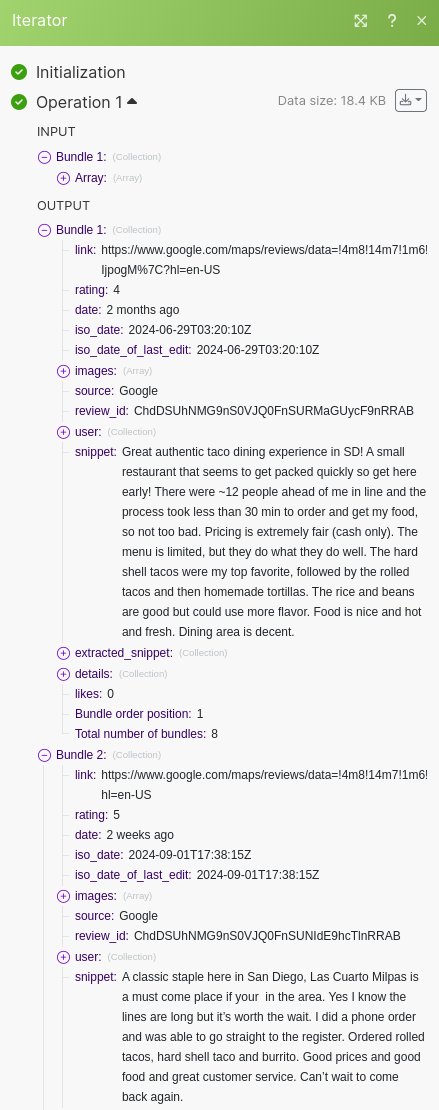
Generate a CSV of Reviews
We've got our data ready to go, but now we need to dump it somewhere for consumption. We'll put it all in a CSV using the aptly named Create CSV Module.
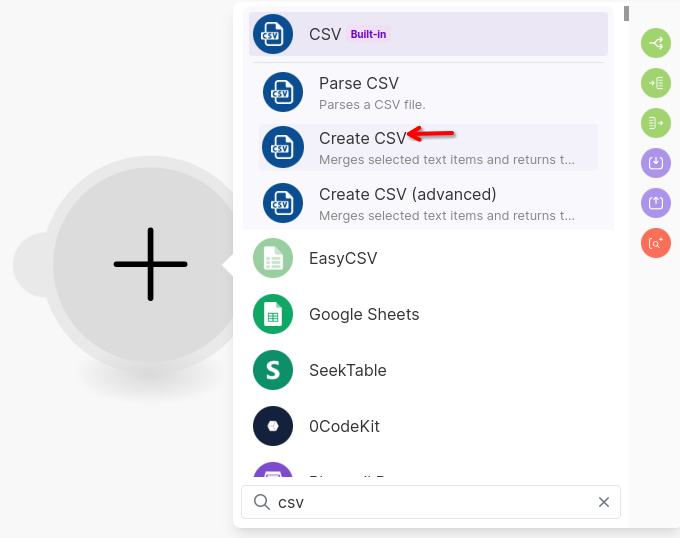
The interface for field selection is a little tricky because we need to select fields from multiple Modules.
First, set the "Source Module" to our first Module "SerpApi - Search Google Maps [1]".
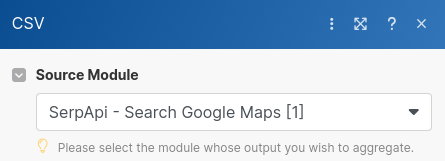
Then under "Aggregated Fields", you'll see a long list of fields organized by which Module they come from. You can select whichever fields you want. For the sake of this tutorial, we want the following data:
- The review rating
- The date of the review
- The review text
- The name of the taqueria
From the second Iterator which is named "Iterator [4]" select the following fields:
rating
iso_date
snippet
(this is the review text)
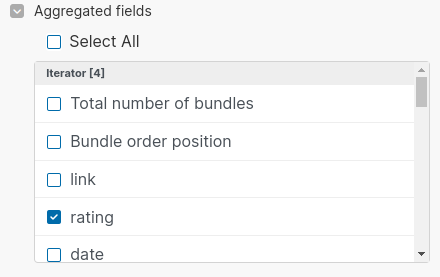
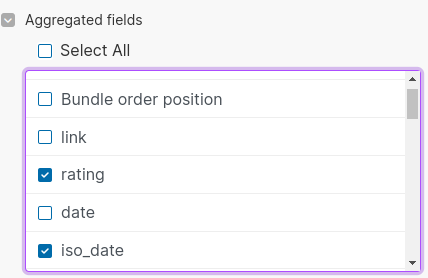
Don't forget snippet
which requires a bit more scrolling.
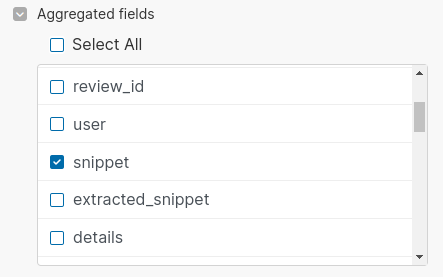
Continue to scroll until you get to the section for "Iterator [2]". This is the first Iterator containing the Google Maps iterated data. We'll select title
from this section which contains the name of the business.
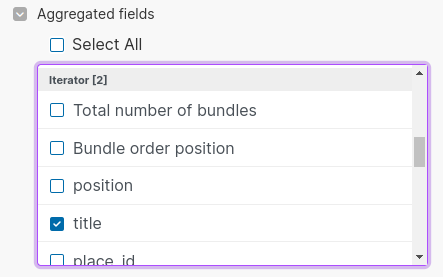
Finally, we'll make sure we're including headers in the first row and the "Delimiter" is set to "Comma."
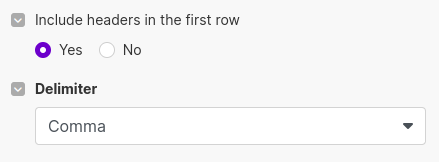
Run the Scenario to confirm we selected the right fields.
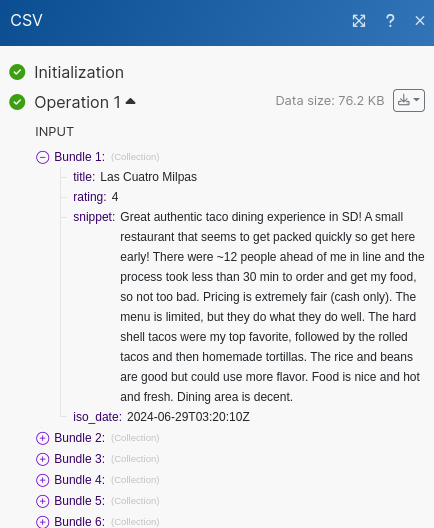
Upload the CSV to Google Drive
We now need a place to put this CSV so we'll upload it to Google Drive using the Upload a File Module in the Google Drive App.
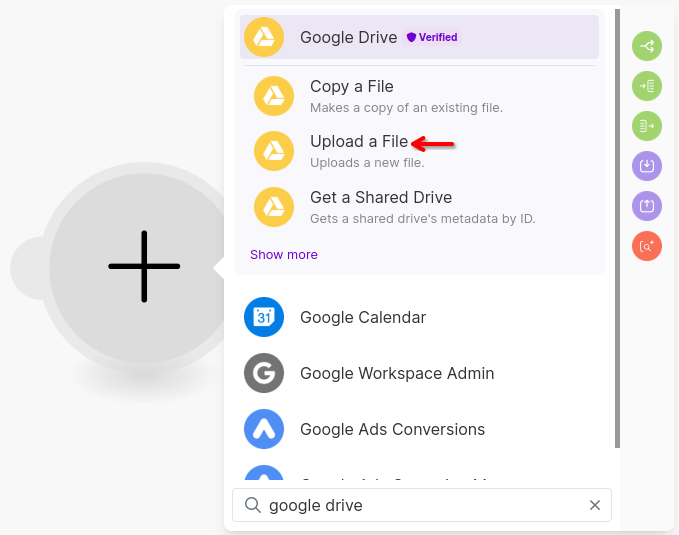
Use an existing Google Connection or create one.
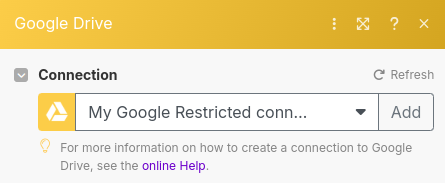
Configure where you'd like the file to be saved in your Google Drive. In the example below, I'm using the root directory of My Drive.
Set a descriptive "File Name" like taqueria_reviews_san_diego.csv
.
And lastly, select the text
output from the Create CSV Module for the "Data" form. This is the raw CSV data.
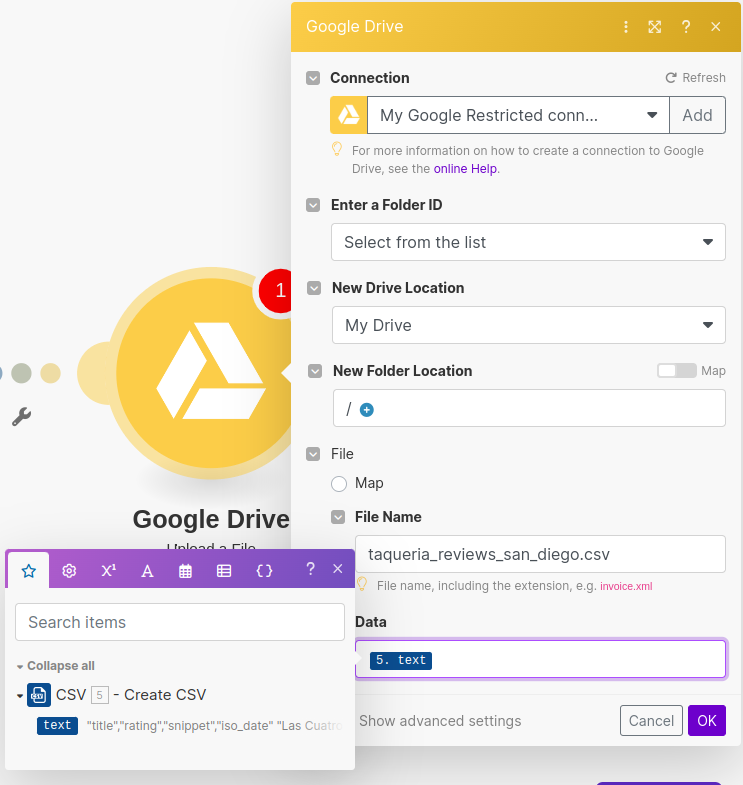
Run the Scenario and it should create a CSV and upload it to your Google Drive.
Check your Google Drive and make sure you have a file that looks like this:
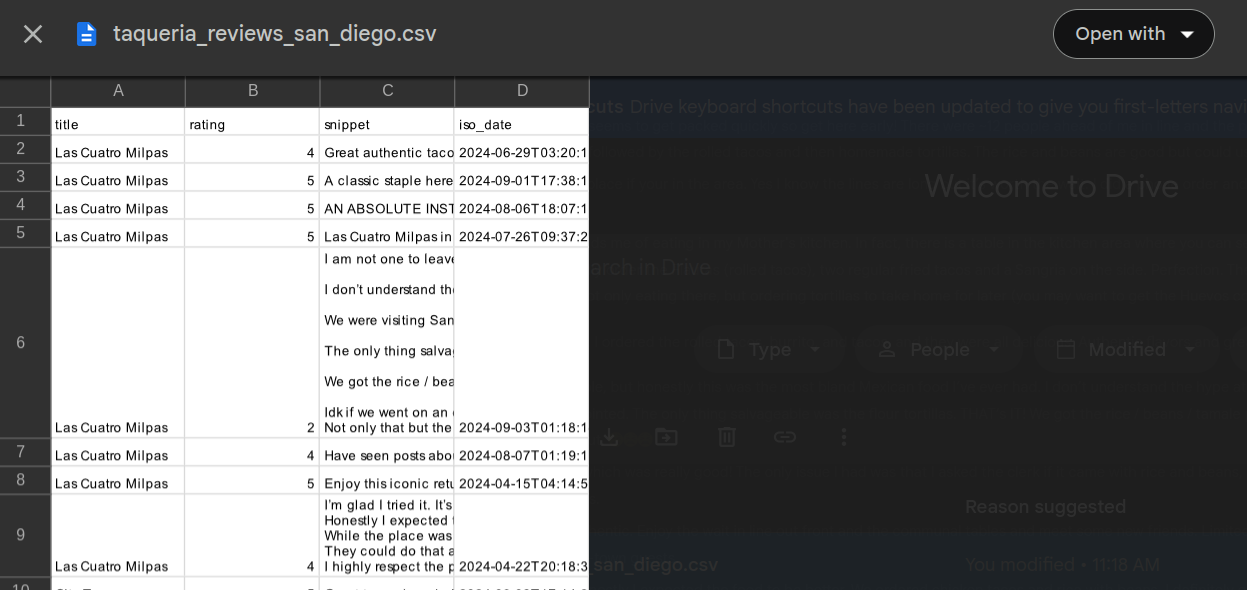
You can also follow the "Open with" button to open the CSV in Google Sheets.
Looks good!
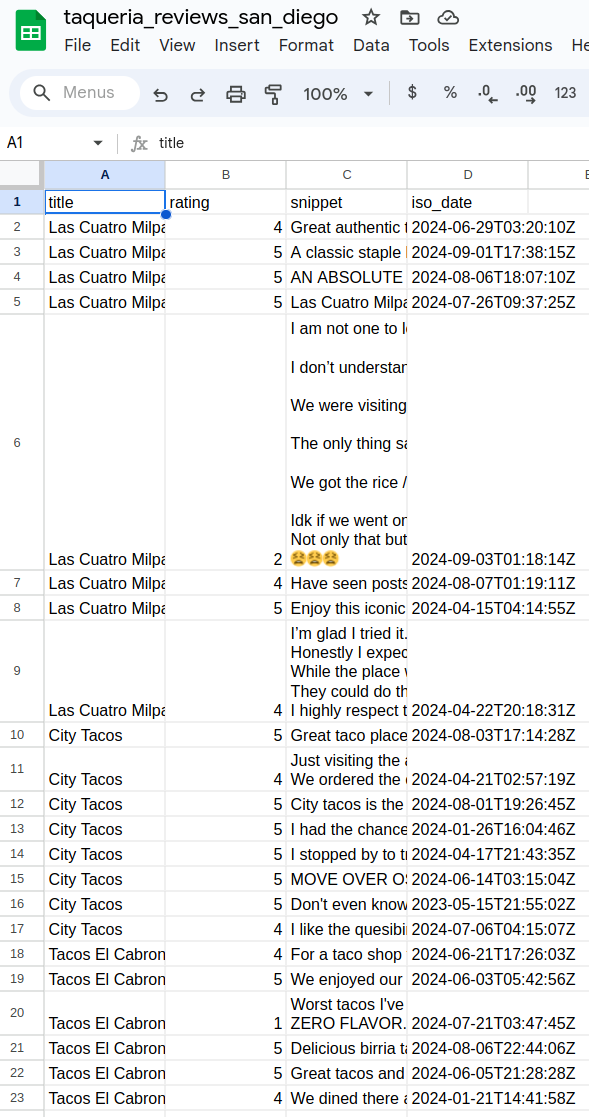
If you'd like to add the data directly to an existing Google Sheet instead of a CSV, please see my guide here:
Add Pagination
As you can see from the screenshot above, we only ended up with 8 reviews per taqueria. And if you recall from the Google Maps section, we only fetched 20 taquerias from San Diego. Surely there are more taquerias and reviews available.
To get these extra taquerias and reviews, we'll need to use pagination. Before getting into that, I should briefly mention that adding pagination will significantly increase the amount of Operations the Make Scenario will use.
Each Make plan has a limit on how many Operations you can run per month. The free plan includes 1,000 Operations per month. If you exceed that, you can no longer run your Scenarios until your free plan renews or you upgrade to a paid plan.
So far our Scenario uses 44 Operations each time it runs. You can see this by looking at the Scenario's History.
You can also add up all the numbers in bubbles linked to each Module.
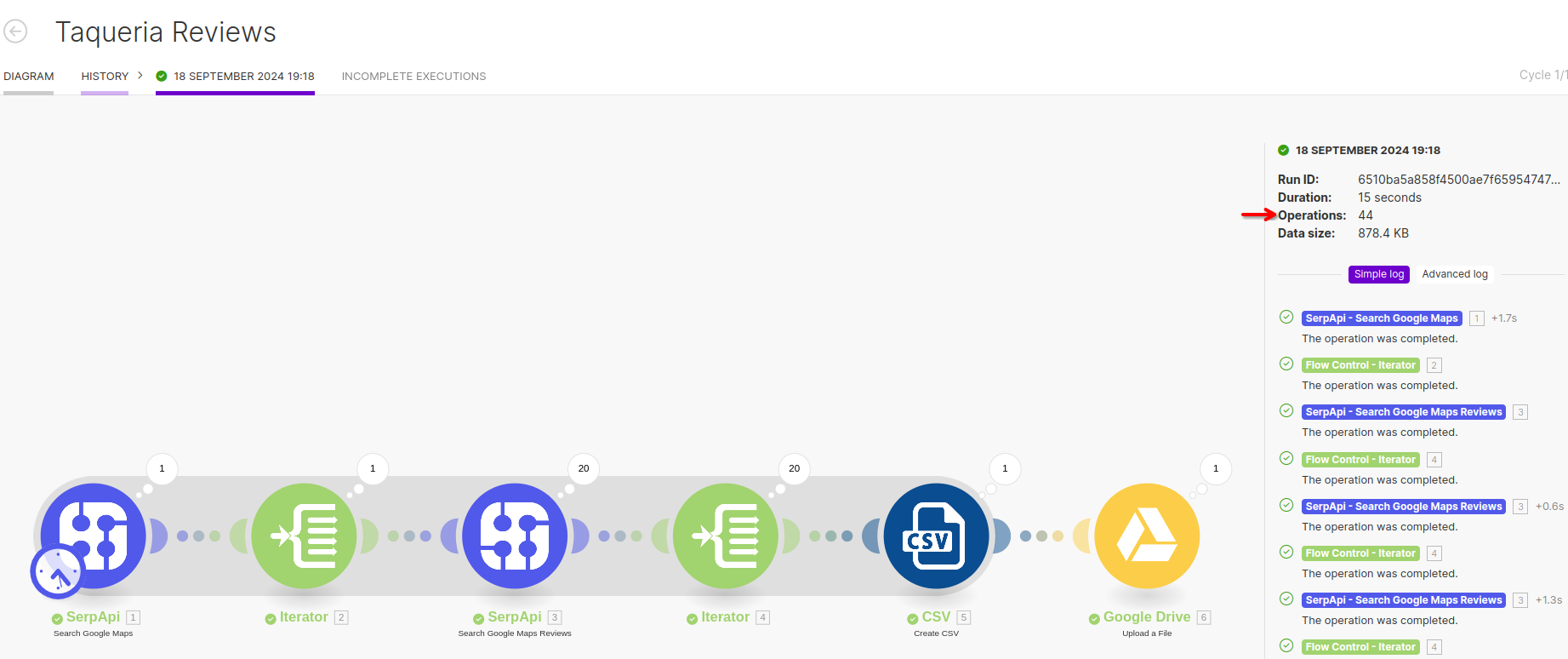
If we add pagination to fetch one extra page of taquerias and each page contains 20 taquerias, that means we'll need to run 40 queries through the Search Google Maps Reviews Module instead of 20. We'll also need to iterate over 40 batches of reviews instead of 20. That will balloon our Operations count from 44 to 84.
And then if we want additional pages of reviews, that will further increase our Operations over 100 per Scenario execution.
Anyway, I just wanted to call this out as something to consider before you decide to add pagination.
Implementing pagination for the Search Google Maps Module is quite simple. Click on the Module and scroll to the bottom of the form and increment the "Pagination Limit" field from 1 to however many pages you want.
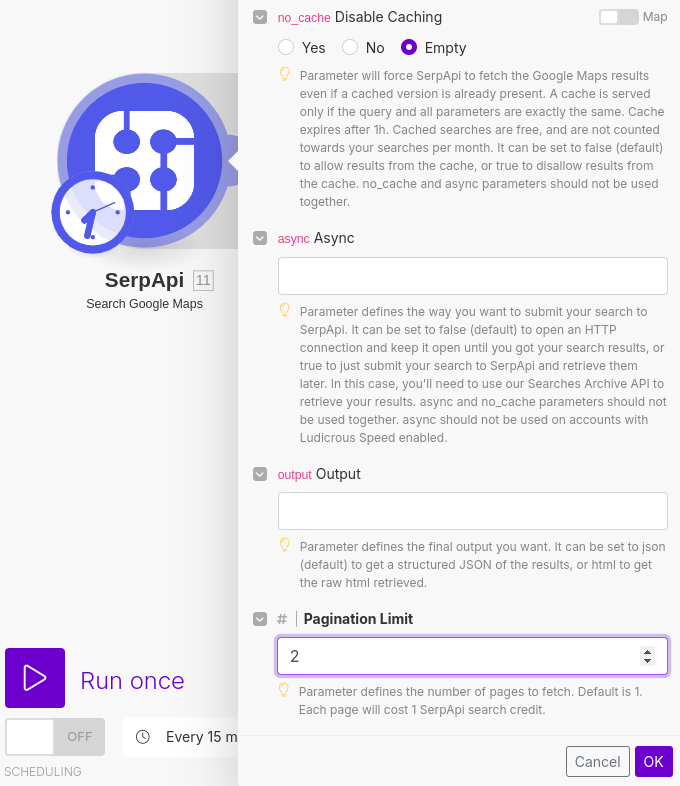
You can then do the same thing for the Search Google Maps Reviews Module.
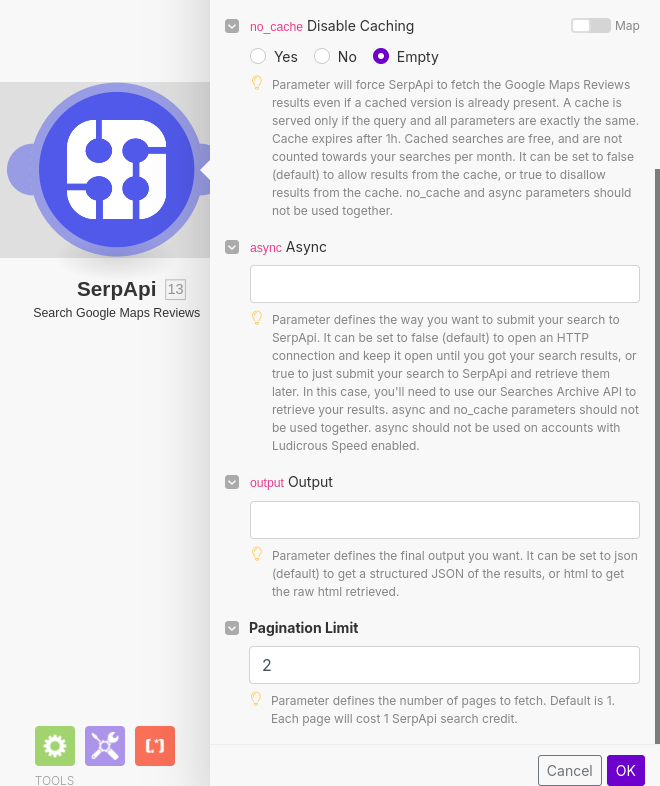
Everything else is automatically handled by Make and the SerpApi Module.
Want to Import This?
If you'd like to skip all this manual configuration yourself, you can download the blueprint of this Scenario and then import it into an empty Scenario. All you'll need to do is configure your own SerpApi and Google connections.