When you search on Google or Google Maps, Google will give a list of businesses, services and points of interest in a specific location.
In my previous post, we learned how to generate a thousand leads when searching for businesses with Google Maps API.
SerpApi also provides high-quality Google Local APIs to scrape all local results.
By scraping Google Local results, you can build rich data of local businesses, including their name, addresses, phone numbers, business hours, websites and customer reviews.
You can also have user reviews, ratings, photos, etc. With this information, you can analyze competitor strategies, optimize local SEO, monitor customer sentiment, improve online presence, and more!
Scrape your first Google Local results with SerpApi
Head to the Google Local Results documentation on SerpApi for details.
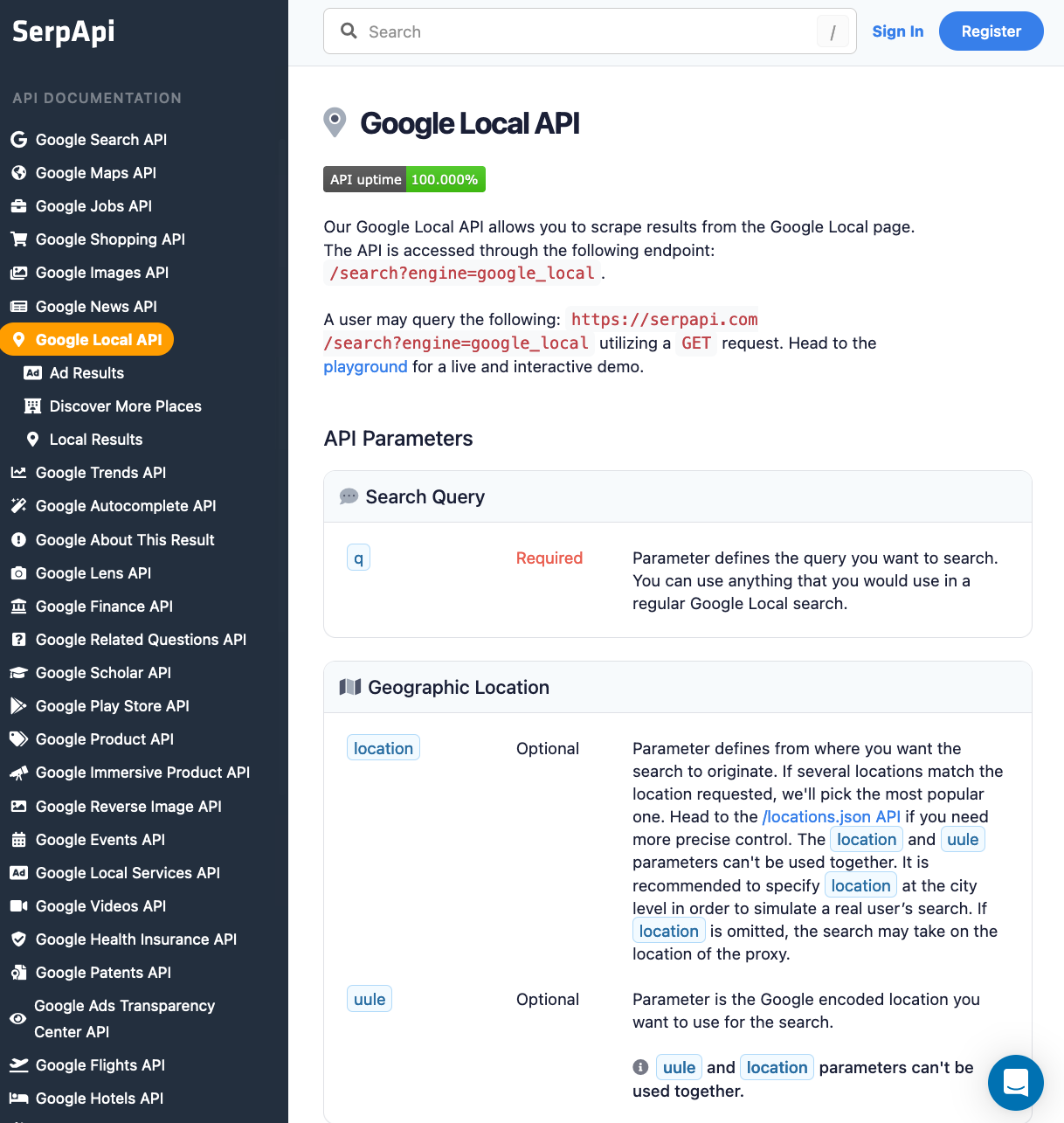
In this tutorial, we will scrape and extract the organic results for all "coffee" stores. The data contains: "position", "rating", "reviews", "place_id", "thumbnail", "title", "type", "address", "hours" and more. You can also scrape more information with SerpApi.
First, you need to install the SerpApi client library.
pip install google-search-results
Set up the SerpApi credentials and search.
import serpapi, os, json
params = {
'api_key': 'YOUR_API_KEY', # your serpapi api
'engine': 'google_local', # SerpApi search engine
'q': 'coffee'
}
To retrieve Google Local Results for a given search query, you can use the following code:
client = serpapi.Client()
results = client.search(params)['local_results']
You can store Google Local Results JSON data in databases or export them to a CSV file.
import csv
header = ['position', 'rating', 'reviews', 'place_id', 'thumbnail', 'title', 'type', 'address', 'hours']
with open('google_local.csv', 'w', encoding='UTF8', newline='') as f:
writer = csv.writer(f)
writer.writerow(header)
for item in results:
print(item)
writer.writerow([item.get('position'), item.get('rating'), item.get('reviews'), item.get('place_id'), item.get('thumbnail'), item.get('title'), item.get('type'), item.get('address'), item.get('hours')])
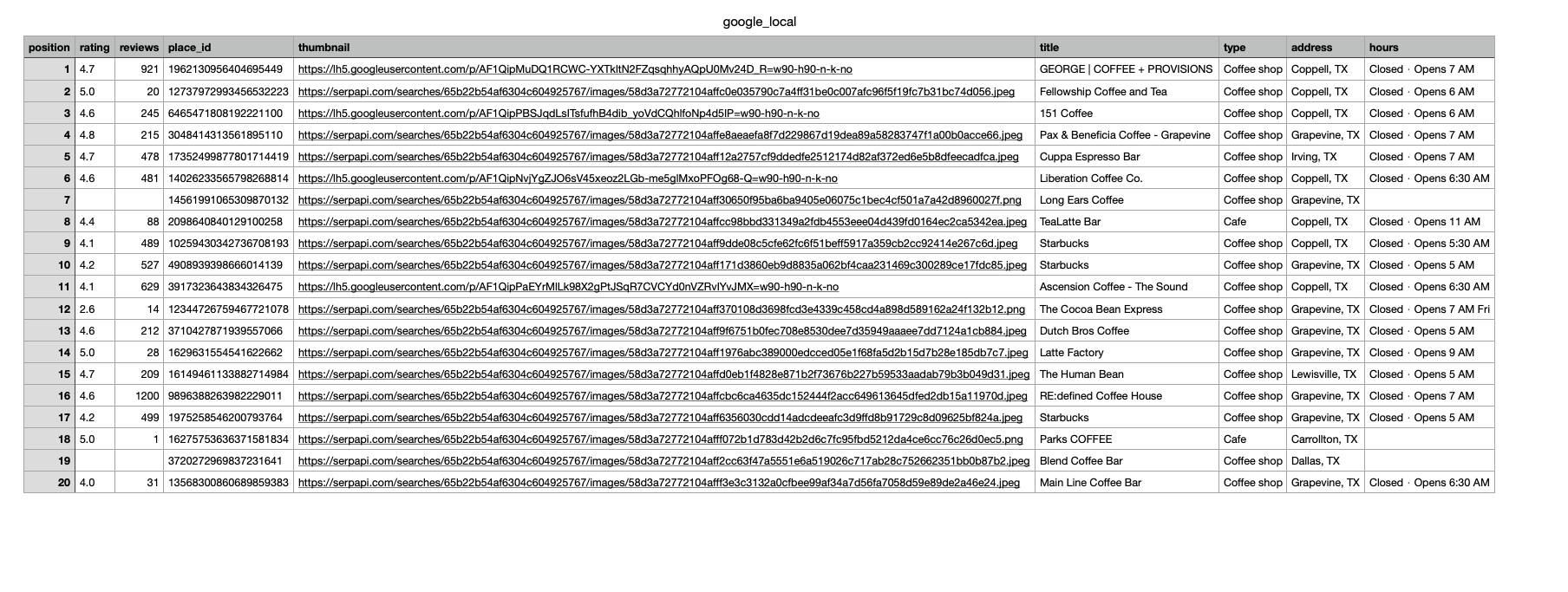
This example is using Python, but you can use your favorite programming languages like Ruby, NodeJS, Java, PHP, etc.
If you have any questions, please feel free to contact me.