Why SerpApi?
Usually, to scrape Google Events you would have to write a tedious long DIY solution using some tools like BeautifulSoup, Puppeteer, Requests, etc. When you use SerpApi, you can offload all the scraping work to us and simply use our APIs to get all the data you need. We have a vast network of proxies running the requests and also use necessary captcha solvers. These are required to avoid getting blocked, and would add a lot of complexity if you try to implement your own solution.
SerpApi offers a structured and dependable method for accessing Google search results without the need for directly scraping Google. Acting as an intermediary, SerpApi manages the intricacies of scraping and returns structured JSON results. This allows you to save time and effort by avoiding the need to build your own Google scraper or rely on other web scraping tools.
Compared to our competitors, we offer superior speed and success rates and, we are able to collect more details from search results than any other provider. We are dedicated to providing the most performant APIs, a legal shield protecting your right to scrape public data, and premium customer service.
About Our Google Events API
The Google Events API allows a user to scrape events from the Google Events page. SerpApi is able to make sense of the information presented on the Google Events page and extract title
, date
, address
, link
, ticket_info
, venue
, thumbnail
and more details about events.
To get started, you can try these searches: "best festivals" or "family friendly events" on our playground. You can also add a location to your search query for more specific searches. For example, "concerts in Chicago".
Here is what you can scrape from the Google Events page:
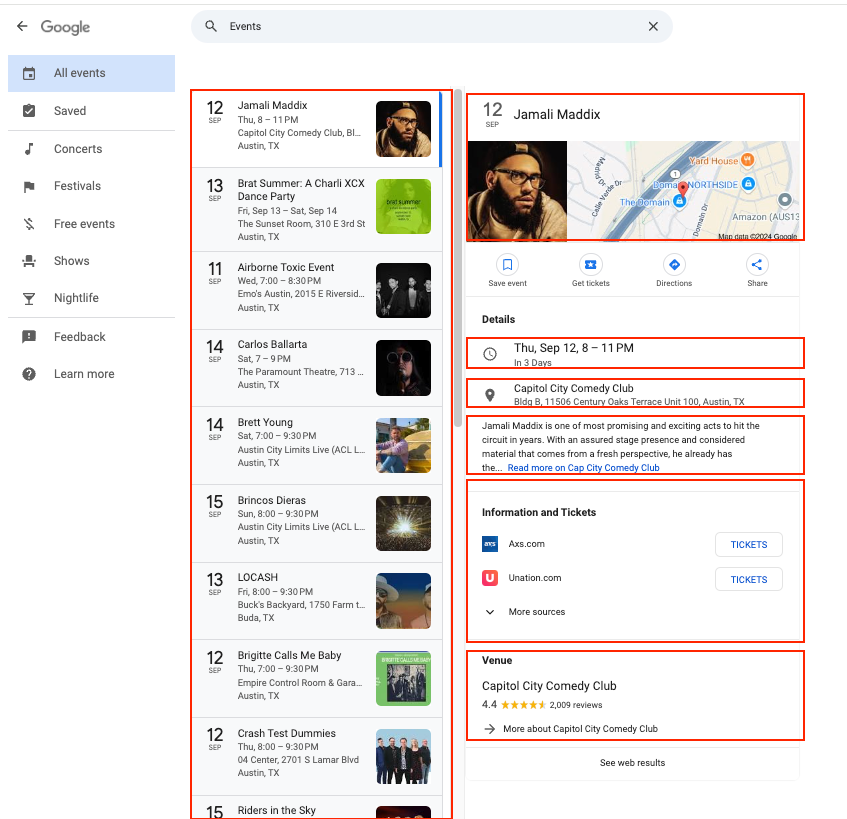
Getting Started With The Google Events API
To begin extracting Google Events data, first, create a free account on serpapi.com. You'll receive one hundred free search credits each month to explore the API.
- Get your SerpApi API Key from this page.
- [Optional but Recommended] Set your API key in an environment variable, instead of directly pasting it in the code. Refer here to understand more about using environment variables. For this tutorial, I have saved the API key in an environment variable named '
API_KEY
'. - Next, on your local computer, you need to install the
google-search-results
Python library:pip install google-search-results
As a side note: You can use this library to scrape search results from other Google Search pages and other search engines, not just Google Events.
Then head to a code editor, and import the necessary libraries to use the Google Events API:
from serpapi import GoogleSearch
import os, json
Following this, we can construct a search query and add the necessary parameters for making a request to the API:
params = {
# https://docs.python.org/3/library/os.html#os.getenv
'api_key': os.getenv('API_KEY'), # your serpapi api
'q': 'Events in Austin', # search query
'engine': 'google_events', # SerpApi search engine
'hl': 'en', # language
'gl': 'us', # country of the search
'start': 0 # pagination
}
The expected response from the API is JSON formatted information on events relevant to the search query. SerpApi's Google Events API is able to extract title
, date
, address
, link
, ticket_info
, venue
, thumbnail
and more.
The response is comprised of four parts:
search_metadata
: This contains the basic data about the search such assearch_id
,status
, endpoint details, timestamps, and more.search_parameters
: This specifies which API parameters have been selected for this particular search.search_information
: This contains extra information about the search such as if Google autocorrected a search query, and more.events_results
: A list of events scraped from Google Events corresponding to the search parameters.
Let's get the results from the API now. Since all the event details are in a list within the events_results
key in the JSON response, let's write some code to get that list:
search = GoogleSearch(params)
results = search.get_dict()
google_events_results = results["events_results"]
The entire code file looks like this:
from serpapi import GoogleSearch
import os, json
params = {
# https://docs.python.org/3/library/os.html#os.getenv
'api_key': os.getenv('API_KEY'), # your serpapi api
'q': 'Events in Austin', # search query
'engine': 'google_events', # SerpApi search engine
'hl': 'en', # language
'gl': 'us', # country of the search
'start': 0 # pagination
}
search = GoogleSearch(params)
results = search.get_dict()
google_events_results = results["events_results"]
The resulting google_events_results
list will look something like this:
[
{
"title": "Open Mic Event",
"date": {
"start_date": "Sep 9",
"when": "Sep 1, 7 PM – Oct 1, 2 AM"
},
"address": ["The Water Tank, 7309 McNeil Dr", "Austin, TX"],
"link": "https://www.openmicaustin.com/MoreInfo/NDk0OCMjIyNURVhU",
"event_location_map": {
"image": "https://www.google.com/maps/vt/data=2iUNKQGKrw3GNEz8OUiTc6nGQvXYjJ6S0rspA4s0ACYjfllywq2B-VeGghoL7g4ALzVliGyjFnE0DIKiXSrtToYXqzL31afVWK90mqOvC2H-S98NjWQ",
"link": "https://www.google.com/maps/place//data=!4m2!3m1!1s0x8644ccdf7d6a2d0f:0x850e4869e28806ef?sa=X&ved=2ahUKEwjru6bJtraIAxVdVTABHenmAbIQ9eIBegQIARAA&hl=en&gl=us",
"serpapi_link": "https://serpapi.com/search.json?data=%214m2%213m1%211s0x8644ccdf7d6a2d0f%3A0x850e4869e28806ef&engine=google_maps&google_domain=google.com&hl=en&q=Events+in+Austin&start=0&type=place"
},
"ticket_info": [
{
"source": "Austin",
"link": "https://www.openmicaustin.com/MoreInfo/NDk0OCMjIyNURVhU",
"link_type": "more info"
}
],
"venue": {
"name": "The Water Tank",
"rating": 4.3,
"reviews": 817,
"link": "https://www.google.com/search?sca_esv=cc60acfe50cff9ff&sca_upv=1&hl=en&gl=us&q=The+Water+Tank&ludocid=9587680276327696111&ibp=gwp%3B0,7"
},
"thumbnail": "https://www.google.com/maps/vt/data=2iUNKQGKrw3GNEz8OUiTc6nGQvXYjJ6S0rspA4s0ACYjfllywq2B-VeGghoL7g4ALzVliGyjFnE0DIKiXSrtToYXqzL31afVWK90mqOvC2H-S98NjWQ"
},
...
...
...
]
An alternate way to build the request is by heading to our playground, and searching with the relevant parameter values, and then using the 'Export to Code' option as demonstrated below:
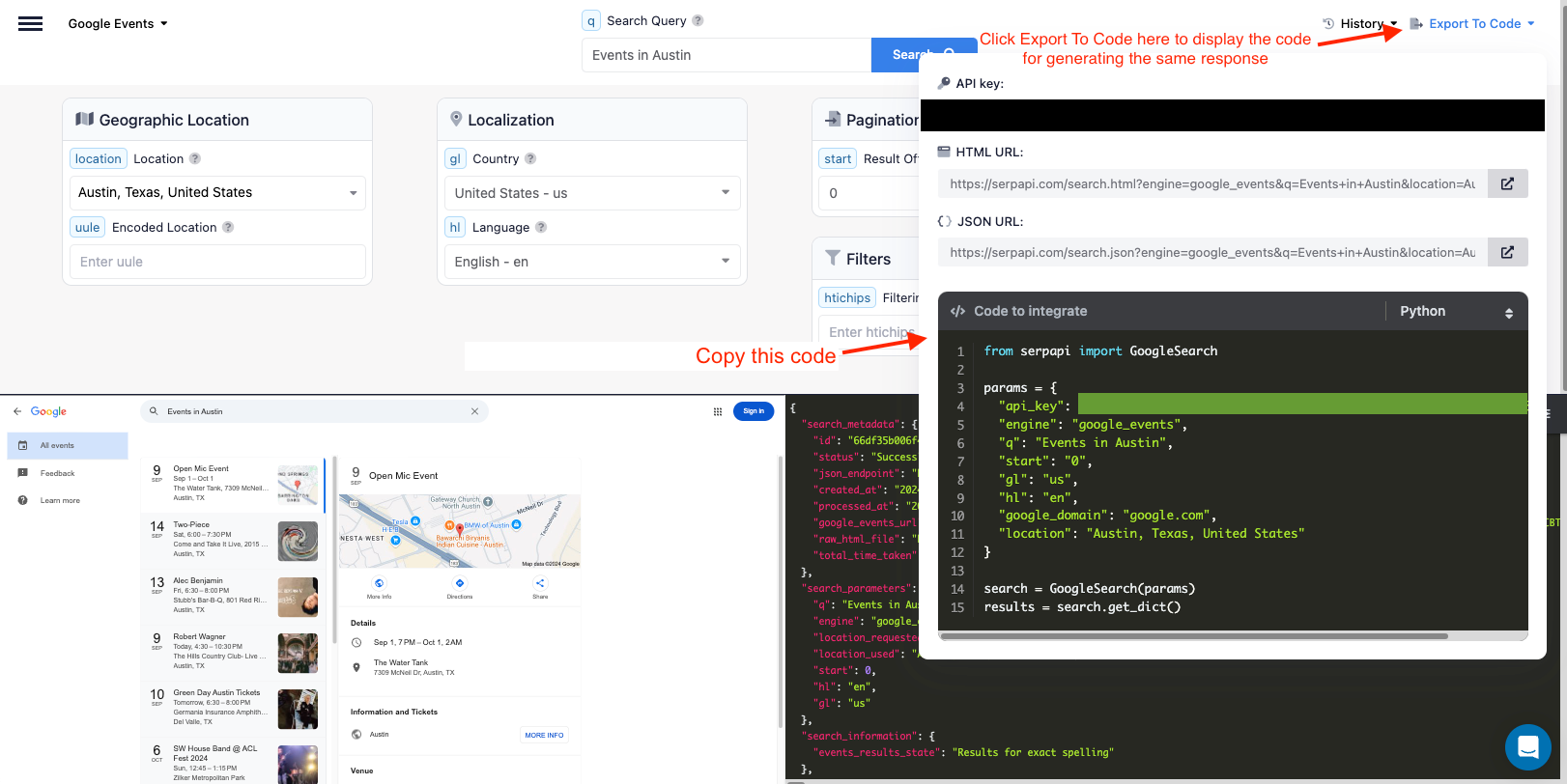
More Information About Our Python Libraries
We have two separate Python libraries serpapi
and google-search-results
, and both work perfectly fine. However, serpapi
is a new one, and all the examples you can find on our website are from the old one google-search-results
. If you'd like to use our Python library with all the examples from our website, you should install the google-search-results
module instead of serpapi
.
For this blog post, I am using google-search-results
because all of our documentation references this one.
You may encounter issues if you have both libraries installed at the same time. If you have the old library installed and want to proceed with using our new library, please follow these steps:
- Uninstall
google-search-results
module from your environment. - Make sure that neither
serpapi
norgoogle-search-results
are installed at that stage. - Install
serpapi
module, for example with the following command if you're usingpip
:pip install serpapi
Setting The Origin Of The Search With Location Specific Parameters
The location
parameter defines where you want the search to originate from. If several locations match the location requested, we'll pick the most popular one. You can pick a location from the available ones at /locations.json API if you need more precise control. It is recommended to specify location at the city level in order to simulate a real user’s search. If location is omitted, the search may take on the location of the proxy.
An alternative to using the location
parameter is using the uule
parameter. This parameter is the Google encoded location you want to use for the search. Please note: The uule
and location
parameters can’t be used together.
Here is an example of using the location
parameter:
params = {
# https://docs.python.org/3/library/os.html#os.getenv
'api_key': os.getenv('API_KEY'), # your serpapi api
'q': 'Events', # search query
'engine': 'google_events', # SerpApi search engine
'hl': 'en', # language
'gl': 'us', # country of the search
'start': 0, # pagination
'location': 'Austin, Texas, United States' # location
}
Playground Example: https://serpapi.com/playground?engine=google_events&q=Events&location=Austin%2C+Texas%2C+United+States&gl=us&hl=en&start=0
Alternatively, you can use the UULE for Austin, Texas, United States:
params = {
# https://docs.python.org/3/library/os.html#os.getenv
'api_key': os.getenv('API_KEY'), # your serpapi api
'q': 'Events', # search query
'engine': 'google_events', # SerpApi search engine
'hl': 'en', # language
'gl': 'us', # country of the search
'start': 0, # pagination
'uule': 'w+CAIQICISQXVzdGluLCBUZXhhcywgVVNB' # location
}
Playground Example: https://serpapi.com/playground?engine=google_events&q=Events&uule=w%2BCAIQICISQXVzdGluLCBUZXhhcywgVVNB&gl=us&hl=en&start=0
This will give you events relevant to the specified location or UULE.
Ways To Get Precise Event Results
In many cases, you may be looking for precise results over a certain time period or for certain types of events. For those cases, I want to highlight some ways to filter event results, and general tips which can help.
Filter Results By Date/Time Specific Parameters
The available options for the htichips
parameter can help filter results by date/time:
date:today
- Today's Eventsdate:tomorrow
- Tomorrow's Eventsdate:week
- This Week's Eventsdate:next_week
- Next Week's Eventsdate:month
- This Month's Eventsdate:next_month
- Next Month's Events
Here is an example of using this parameter to get results for today's events:
params = {
# https://docs.python.org/3/library/os.html#os.getenv
'api_key': os.getenv('API_KEY'), # your serpapi api
'q': 'Events in Austin', # search query
'engine': 'google_events', # SerpApi search engine
'hl': 'en', # language
'gl': 'us', # country of the search
'start': 0, # pagination
'location': 'Austin, Texas, United States', # location
'htichips': 'date:today' # filter by date
}
Playground Example: https://serpapi.com/playground?engine=google_events&q=Events+in+Austin&location=Austin%2C+Texas%2C+United+States&gl=us&hl=en&start=0&htichips=date%3Atoday
This will give you events happening today in Austin, Texas, United States.
'htichips':'date:today,date:tomorrow'
will give you results for today's and tomorrow's events.Tips To Use The Query Parameter To Get Precise Results
Every request has the required query parameter q
which gets you relevant events. You can word your query in certain ways to get more precise results.
To get results for events happening next year in Austin, Texas, you can form your query parameter like this:
'q' = 'Events in Austin in 2025'
Playground Example: https://serpapi.com/playground?engine=google_events&q=Events+in+Austin+in+2025&location=Austin%2C+Texas%2C+United+States&gl=us&hl=en&start=0
To get results for events related to music happening this year, you can form your query parameter like this:
'q' = 'Music related Events in 2024'
Playground Example: https://serpapi.com/playground?engine=google_events&q=Music+related+Events+in+2024&location=Austin%2C+Texas%2C+United+States&gl=us&hl=en&start=0
To get results for events related to sports happening within 2 particular months of 2024, you can form your query parameter like this:
'q' = 'Sports Related Events in October and November 2024'
Playground Example: https://serpapi.com/playground?engine=google_events&q=Sports+Related+Events+in+October+and+November+2024&location=Austin%2C+Texas%2C+United+States&gl=us&hl=en&start=0
For all the examples above I've still specified the location parameter as Austin, Texas, United States because if the location is omitted, the search may take on the location of the proxy. You can specify it in your code like this:
'location': 'Austin, Texas, United States'
Just like the examples above, you can modify the query in many different ways and get relevant results for your use case.
Pagination
By default, the Google Events API response returns 10 events at a time.
If you'd like to access more results, you need to paginate using the start parameter. Here are a couple of examples:
1st page of results - First 10 results (use start=0
parameter):
start = 0
by default.2nd page of results - Next 10 results (use start=10
parameter):
https://serpapi.com/playground?engine=google_events&q=Events+in+Austin&location=Austin%2C+Texas%2C+United+States&gl=us&hl=en&start=10
3rd page of results - Next 10 results (use start=20
parameter):
https://serpapi.com/playground?engine=google_events&q=Events+in+Austin&location=Austin%2C+Texas%2C+United+States&gl=us&hl=en&start=20
Conclusion
We've covered how to scrape Google Events with SerpApi's Google Events API using SerpApi's Python library.
I hope you found this tutorial helpful. If you have any questions, don't hesitate to reach out to me at sonika@serpapi.com.
Relevant Links
Documentation
Related Posts
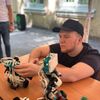
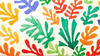