AI Agent is eating the world. The current state-of-the-art LLM has an adequate level of human reasoning. It is exciting because it can help take care of the mundane tasks, and leave us to do the tasks we care about. In this blog post, I will showcase how to create an AI agent using CrewAI and provide the necessary tools for the agent, such as SerpApi, to help build a sports analysis application.
AI Agents are different from ChatGPT. While they may use the same LLM model, their workflows are distinct. In ChatGPT, you ask a question, and it provides an answer. Occasionally, it uses tools to craft a more insightful response. This is all good. And for an AI Agent, it tries to mimic human behavior. They have goals, they have a backstory (like a System Prompt), they reason and reflect and they work their way to achieve the goal. ChatGPT provides answers based on its trained knowledge base. The AI agent conducts research, reasons through the information, and provides an answer once sufficient data has been collected.
We are still in the early stages, though AI Agents are great, they can't escape from hallucination, yet.
Back to building the application, we're building a sport analysis application using LLM, CrewAI, and SerpApi.
CrewAI is an AI Agent framework. It lets you build a team of agents with ease.
SerpApi provides Google search data. Just one API call away to get any data from Google search. It handles the complicated tasks of managing proxies and keeping the data up to date with Google layouts.
The end goal is to produce a report that covers match results, the latest news, and recent transfer updates for a given sports team.
Designing the agents
You can think agent like a team you manage in a company. If you have unlimited resources, how will you delegate tasks to each member? For our application, I think we need 2 agents. Their job scope should be as narrow as possible. The first agent is responsible for researching information. The 2nd agent is responsible for writing the report from the collected information.
Here is how it looks using CrewAI. The code below creates the agent.
researcher = Agent(
role='Senior Sport Researcher',
goal='Retrieve the latest {sport} news including %s on {team}' % (interests_joined),
verbose=True,
memory=True,
backstory=(
"Every morning, the first thing you do are reading the news"
"You often interact with the managers of teams to get the latest information"
"You always think you have the best news in my mind"
"You always has the insider information"
),
tools=[search_tool],
allow_delegation=True
)
Research Agent
writer = Agent(
role='Enthusiastic Writer',
goal='Narrate compelling {sport} news about {team}',
verbose=True,
memory=True,
backstory=(
"With a flair for simplifying complex topics, you craft"
"engaging narratives that captivate and educate, bringing new"
"discoveries to light in an accessible manner."
),
tools=[search_tool],
allow_delegation=False
)
Writing Agent
The search_tool
is using SerpApi under the hood. The code is shared later in the blog post.
Defining the tasks
We have created the agents. Now, we need to create the tasks that work towards our goal. Tasks set the direction the agents will take.
The first is researching.
research_task = Task(
description=(
f"Today date: {datetime.now().date()}"
"Identify the latest news in {sport} on {team}."
f"You have to reasearch about {interests_joined}. All the topics listed are important"
"Your final report should clearly articulate the topics listed,"
"the good news for the {team} and the bad news for the {team}."
),
expected_output='A comprehensive 3 paragraphs long report on {team}.',
tools=[search_tool],
agent=researcher,
)
And obviously, the second task is writing.
write_task = Task(
description=(
"Compose an enthusiastic and insightful article on {team}."
"Focus on the latest news and how it's impacting the {sport}."
"This article should be easy to understand, engaging, and positive."
),
expected_output='A 4 paragraph article on {team}. And a title for the article enclosing with ###',
tools=[search_tool],
agent=writer,
async_execution=False,
callback=handle_output
)
Assemble
We are ready to bring everything together and start doing the work.
crew = Crew(
agents=[researcher, writer],
tasks=[research_task, write_task],
process=Process.sequential,
memory=True,
cache=True,
max_rpm=100,
share_crew=True
)
result = crew.kickoff(inputs={'sport': 'Soccer', 'team': 'Manchester City'})
The call to kickoff
starts the process.
Full code
from dotenv import load_dotenv
load_dotenv()
import re
import os
from crewai import Agent, Task, Crew, Process
from serpapi_google_search_tool import SerpApiGoogleSearchTool
from datetime import datetime
# os.environ["OPENAI_MODEL_NAME"]="gpt-4o"
os.environ["OPENAI_MODEL_NAME"]="gpt-4-turbo"
search_tool = SerpApiGoogleSearchTool()
interests = [
'Match results. Scores, player who score, the ranking changes are important',
'Latest news about the club',
'Transfer news'
]
interests_joined = ', '.join(interests)
researcher = Agent(
role='Senior Sport Researcher',
goal='Retrieve the latest {sport} news including %s on {team}' % (interests_joined),
verbose=True,
memory=True,
backstory=(
"Every morning, the first thing you do are reading the news"
"You often interact with the managers of teams to get the latest information"
"You always think you have the best news in my mind"
"You always has the insider information"
),
tools=[search_tool],
allow_delegation=True
)
writer = Agent(
role='Enthusiastic Writer',
goal='Narrate compelling {sport} news about {team}',
verbose=True,
memory=True,
backstory=(
"With a flair for simplifying complex topics, you craft"
"engaging narratives that captivate and educate, bringing new"
"discoveries to light in an accessible manner."
),
tools=[search_tool],
allow_delegation=False
)
research_task = Task(
description=(
f"Today date: {datetime.now().date()}"
"Identify the latest news in {sport} on {team}."
f"You have to reasearch about {interests_joined}. All the topics listed are important"
"Your final report should clearly articulate the topics listed,"
"the good news for the {team} and the bad news for the {team}."
),
expected_output='A comprehensive 3 paragraphs long report on {team}.',
tools=[search_tool],
agent=researcher,
)
def handle_output(output):
content = output.raw_output
pattern = r"###(.*?)###"
match = re.search(pattern, content)
filename = 'new-article.md'
if match:
filename = f'{match.group(1)}.md'
with open(filename, "w") as file:
file.write(content)
write_task = Task(
description=(
"Compose an enthusiastic and insightful article on {team}."
"Focus on the latest news and how it's impacting the {sport}."
"This article should be easy to understand, engaging, and positive."
),
expected_output='A 4 paragraph article on {team}. And a title for the article enclosing with ###',
tools=[search_tool],
agent=writer,
async_execution=False,
callback=handle_output
)
crew = Crew(
agents=[researcher, writer],
tasks=[research_task, write_task],
process=Process.sequential,
memory=True,
cache=True,
max_rpm=100,
share_crew=True
)
result = crew.kickoff(inputs={'sport': 'Soccer', 'team': 'Manchester City'})
# serpapi_google_search_tool.py
import os
import requests
from typing import Type, Any
from pydantic.v1 import BaseModel, Field
from crewai_tools.tools.base_tool import BaseTool
class SerpApiGoogleSearchToolSchema(BaseModel):
q: str = Field(..., description="Parameter defines the query you want to search. You can use anything that you would use in a regular Google search. e.g. inurl:, site:, intitle:.")
class SerpApiGoogleSearchTool(BaseTool):
name: str = "Google Search"
description: str = "Search the internet"
args_schema: Type[BaseModel] = SerpApiGoogleSearchToolSchema
search_url: str = "https://serpapi.com/search"
def _run(
self,
q: str,
**kwargs: Any,
) -> Any:
payload = {
"engine": "google",
"q": q,
"api_key": os.getenv('SERPAPI_API_KEY'),
}
headers = {
'content-type': 'application/json'
}
response = requests.request("GET", self.search_url, headers=headers, params=payload)
results = response.json()
summary = ""
if 'answer_box_list' in results:
summary += str(results['answer_box_list'])
elif 'answer_box' in results:
summary += str(results['answer_box'])
elif 'organic_results' in results:
summary += str(results['organic_results'])
elif 'sports_results' in results:
summary += str(results['sports_results'])
elif 'knowledge_graph' in results:
summary += str(results['knowledge_graph'])
elif 'top_stories' in results:
summary += str(results['top_stories'])
return summary
Refer to SerpApi's documentation for more information.
Remember to install the CrewAI python package
pip install crewai 'crewai[tools]'
Output
The latest result while crafting this blog post.
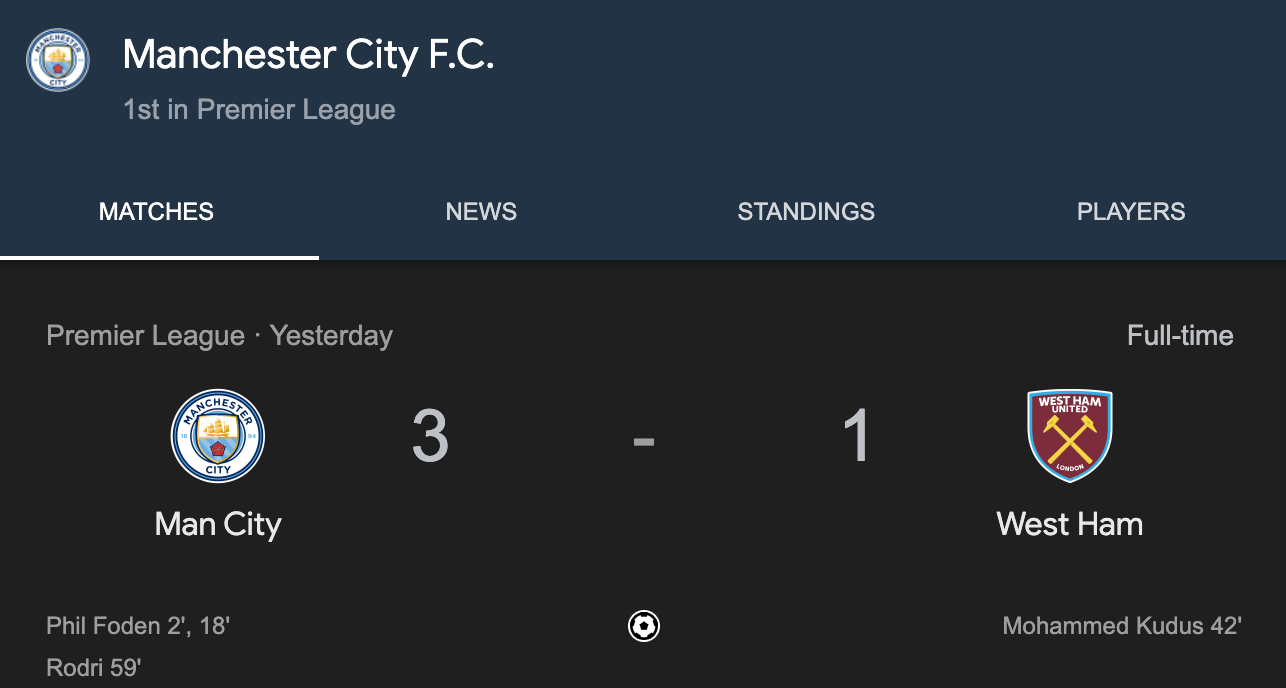
The answer from our agents are comprehensive and it got the information right.
In the grand tapestry of English football, few threads shimmer as brightly as Manchester City's recent conquests. The club's vibrant journey reached another pinnacle as they clinched their fourth consecutive English Premier League title, outplaying West Ham in a thrilling 3-1 victory. With Phil Foden's double strike and Rodri's decisive goal in the second half, the match was not just a display of skill but a statement of dominance. This historic win not only cements their legacy but also showcases the strategic brilliance of Pep Guardiola and the relentless spirit of his squad.
The echoes of victory at Etihad Stadium have reverberated across the football world, affirming Manchester City's top position in the league standings. Their sixth title in the past seven years is a testament to a consistent strategy that melds youthful energy with experienced tact. This era of success underlines a period of well-crafted team dynamics and managerial excellence, which has been instrumental in their sustained dominance. As fans celebrate, analysts and pundits are already heralding this team as one of the all-time greats in Premier League history.
Off the pitch, the club keeps making strategic moves to ensure their future is as bright as their present. Although specific details remain under wraps, the ongoing transfer rumors suggest a keen eye on strengthening the squad further. Manchester City's approach in the transfer market reflects a clear vision to maintain competitiveness and address any potential gaps. This proactive stance in player acquisition and development continues to keep them at the forefront of not just national, but global football relevance.
As Manchester City gears up for the upcoming seasons, their story is far from over. With a blend of tactical genius, a robust squad, and a hunger for more glory, they are not just participating in competitions; they are setting the benchmarks. For fans around the world, Manchester City is not just a club to watch, but a phenomenon to experience. Their journey is a vivid narrative of triumph, ambition, and footballing excellence, promising even more thrilling chapters in the seasons to come.
It is interesting to observe the "thoughts" of the agents:
I need to gather the latest information on Manchester City concerning match results, scores, player performances, ranking changes, general club news, and transfer news. To do this efficiently, I will use Google Search to find the most recent and relevant data.
Action: Google Search
Action Input: {"q": "Manchester City latest match results scores players ranking changes club news transfer news May 2024"}
...
Thought:
I have gathered various snippets that provide the latest information on Manchester City. To construct a comprehensive report addressing match results, scores, player performances, ranking changes, club news, and transfer news, I need to synthesize the data from these snippets.
I will summarize the gathered information into a well-structured report divided into three main sections: Match Results and Performances, Club News and Ranking Changes, and Transfer News.
...
On the other hand, if I ask a similar question in ChatGPT, it seems to have the potential to provide a well-written response, but the information is often inaccurate.
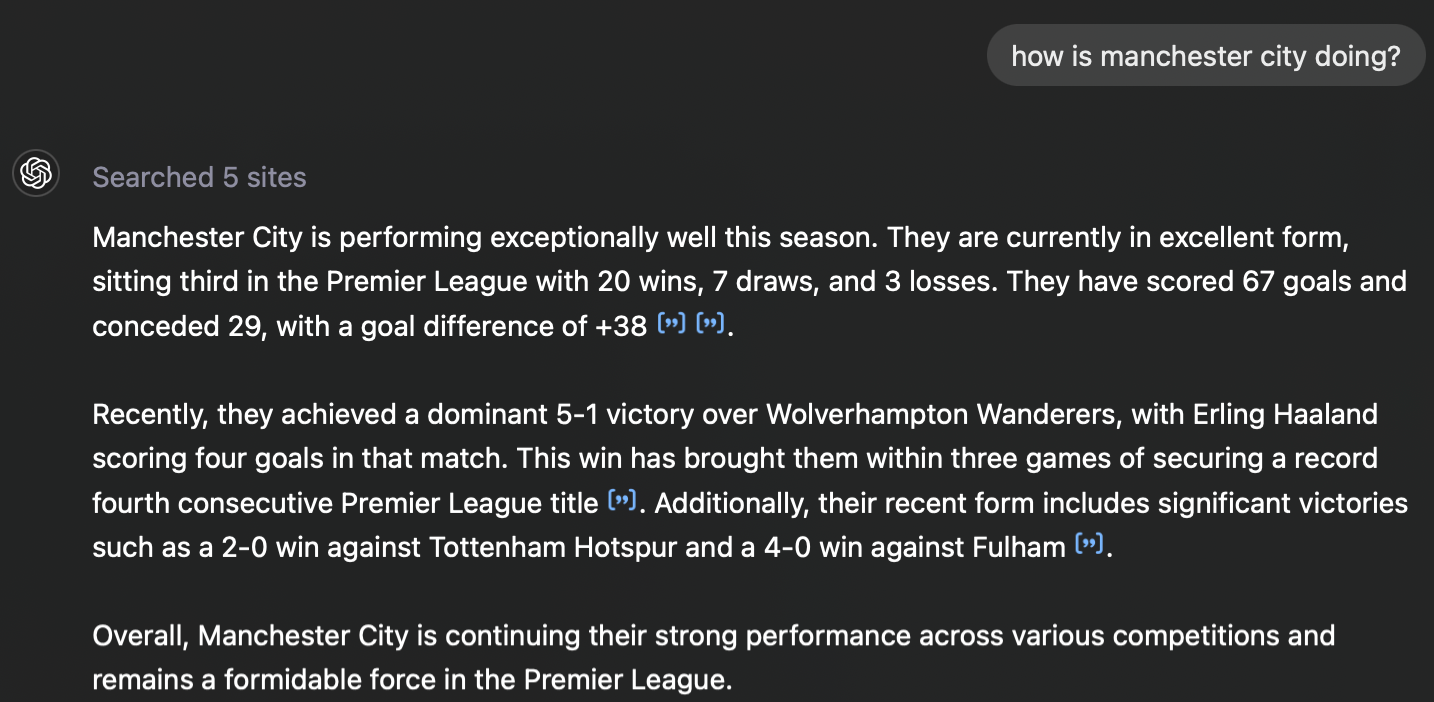
Conclusions
LLMs open the "human reasoning door" in computer which we never have before. Popular AI scientist Andrew Ng also advocate the work on AI Agents. Thus, companies are investing into developing AI Agents. They have great potential to help us tackle mundane tasks. I am only showing a tiny piece of the potential. If you have real business use-cases, I think it is a good opportunity to give it a try.
Thank you for reading and I hope you find it useful. I'll be sharing more AI related articles, please feel free to follow our social media for latest updates.
Add a Feature Request💫 or a Bug🐞